NumPy - 快速指南
NumPy - Introduction
NumPy是一个Python包。 它代表'Numerical Python'。 它是一个由多维数组对象和用于处理数组的例程集合组成的库。
Numeric ,NumPy的祖先,由Jim Hugunin开发。 Numarray的另一个包也开发了,具有一些额外的功能。 2005年,Travis Oliphant通过将Numarray的功能整合到Numeric包中来创建NumPy包。 这个开源项目有很多贡献者。
使用NumPy的操作
使用NumPy,开发人员可以执行以下操作 -
数组的数学和逻辑运算。
用于形状操纵的傅里叶变换和例程。
与线性代数有关的操作。 NumPy具有线性代数和随机数生成的内置函数。
NumPy - MatLab的替代品
NumPy经常与SciPy (Scientific Python)和Mat−plotlib (绘图库)等软件包一起使用。 这种组合被广泛用作MatLab的替代品,MatLab是一种流行的技术计算平台。 但是,Python替代MatLab现在被视为一种更现代和完整的编程语言。
它是开源的,这是NumPy的附加优势。
NumPy - Environment
标准Python发行版不与NumPy模块捆绑在一起。 一个轻量级的替代方案是使用流行的Python包安装程序pip安装NumPy。
pip install numpy
启用NumPy的最佳方法是使用特定于您的操作系统的可安装二进制包。 这些二进制文件包含完整的SciPy堆栈(包括NumPy,SciPy,matplotlib,IPython,SymPy和nose包以及核心Python)。
Windows
Anaconda(来自https://www.continuum.io )是SciPy堆栈的免费Python发行版。 它也适用于Linux和Mac。
Canopy( https://www.enthought.com/products/canopy/ )免费提供商业发行版,并提供适用于Windows,Linux和Mac的完整SciPy堆栈。
Python(x,y):它是一个免费的Python发行版,包含SciPy堆栈和适用于Windows操作系统的Spyder IDE。 (可从https://www.python-xy.github.io/下载)
Linux
各个Linux发行版的软件包管理器用于在SciPy堆栈中安装一个或多个软件包。
对于Ubuntu
sudo apt-get install python-numpy
python-scipy python-matplotlibipythonipythonnotebook python-pandas
python-sympy python-nose
对于Fedora
sudo yum install numpyscipy python-matplotlibipython
python-pandas sympy python-nose atlas-devel
从Source构建
必须使用distutils安装Core Python(2.6.x,2.7.x和3.2.x及更高版本),并且应启用zlib模块。
GNU gcc(4.2及以上版本)C编译器必须可用。
要安装NumPy,请运行以下命令。
Python setup.py install
要测试NumPy模块是否已正确安装,请尝试从Python提示符导入它。
import numpy
如果未安装,将显示以下错误消息。
Traceback (most recent call last):
File "<pyshell#0>", line 1, in <module>
import numpy
ImportError: No module named 'numpy'
或者,使用以下语法导入NumPy包 -
import numpy as np
NumPy - Ndarray Object
NumPy中定义的最重要的对象是名为ndarray的N维数组类型。 它描述了相同类型的项目集合。 可以使用从零开始的索引访问集合中的项目。
ndarray中的每个项目在内存中占用相同大小的块。 ndarray中的每个元素都是数据类型对象的对象(称为dtype )。
从ndarray对象中提取的任何项(通过切片)由一个数组标量类型的Python对象表示。 下图显示了ndarray,数据类型对象(dtype)和数组标量类型之间的关系 -
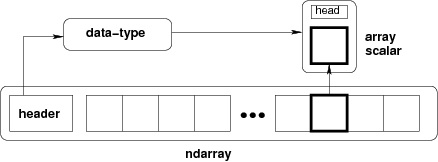
ndarray类的实例可以通过本教程后面描述的不同数组创建例程来构造。 基本的ndarray是使用NumPy中的数组函数创建的,如下所示 -
numpy.array
它从任何暴露数组接口的对象或从任何返回数组的方法创建一个ndarray。
numpy.array(object, dtype = None, copy = True, order = None, subok = False, ndmin = 0)
上面的构造函数采用以下参数 -
Sr.No. | 参数和描述 |
---|---|
1 | object 暴露数组接口方法的任何对象都返回一个数组或任何(嵌套)序列。 |
2 | dtype 期望的数组数据类型,可选 |
3 | copy 可选的。 默认情况下(true),复制对象 |
4 | order C(行主要)或F(列主要)或A(任何)(默认) |
5 | subok 默认情况下,返回的数组强制为基类数组。 如果为true,则子类通过 |
6 | ndmin 指定结果数组的最小尺寸 |
请查看以下示例以更好地理解。
例子1 (Example 1)
import numpy as np
a = np.array([1,2,3])
print a
输出如下 -
[1, 2, 3]
例子2 (Example 2)
# more than one dimensions
import numpy as np
a = np.array([[1, 2], [3, 4]])
print a
输出如下 -
[[1, 2]
[3, 4]]
例子3 (Example 3)
# minimum dimensions
import numpy as np
a = np.array([1, 2, 3,4,5], ndmin = 2)
print a
输出如下 -
[[1, 2, 3, 4, 5]]
例子4 (Example 4)
# dtype parameter
import numpy as np
a = np.array([1, 2, 3], dtype = complex)
print a
输出如下 -
[ 1.+0.j, 2.+0.j, 3.+0.j]
ndarray对象由连续的计算机存储器的一维段组成,并与将每个项映射到存储器块中的位置的索引方案相结合。 内存块以行主要顺序(C样式)或列主要顺序(FORTRAN或MatLab样式)保存元素。
NumPy - Data Types
NumPy支持比Python更多种类的数值类型。 下表显示了NumPy中定义的不同标量数据类型。
Sr.No. | 数据类型和描述 |
---|---|
1 | bool_ 存储为字节的布尔值(True或False) |
2 | int_ 默认整数类型(与C long相同;通常为int64或int32) |
3 | intc 与C int相同(通常为int32或int64) |
4 | intp 用于索引的整数(与C ssize_t相同;通常为int32或int64) |
5 | int8 字节(-128到127) |
6 | int16 整数(-32768至32767) |
7 | int32 整数(-2147483648至2147483647) |
8 | int64 整数(-9223372036854775808至9223372036854775807) |
9 | uint8 无符号整数(0到255) |
10 | uint16 无符号整数(0到65535) |
11 | uint32 无符号整数(0到4294967295) |
12 | uint64 无符号整数(0到18446744073709551615) |
13 | float_ float64的简写 |
14 | float16 半精度浮点数:符号位,5位指数,10位尾数 |
15 | float32 单精度浮点数:符号位,8位指数,23位尾数 |
16 | float64 双精度浮点数:符号位,11位指数,52位尾数 |
17 | complex_ complex128的简写 |
18 | complex64 复数,由两个32位浮点数(实部和虚部)表示 |
19 | complex128 复数,由两个64位浮点数(实数和虚数组件)表示 |
NumPy数字类型是dtype(数据类型)对象的实例,每个对象都具有唯一的特征。 dtypes可用作np.bool_,np.float32等。
Data Type Objects (dtype)
数据类型对象描述对应于数组的固定内存块的解释,具体取决于以下方面 -
数据类型(整数,浮点或Python对象)
数据大小
字节顺序(little-endian或big-endian)
在结构化类型的情况下,每个字段的字段名称,每个字段的数据类型和每个字段占用的内存块的一部分。
如果数据类型是子数组,则其形状和数据类型
字节顺序由前缀“”决定数据类型。 ' '>'表示编码是big-endian(最重要的字节存储在最小的地址中)。
使用以下语法构造dtype对象 -
numpy.dtype(object, align, copy)
参数是 -
Object - 要转换为数据类型对象
Align - 如果为true,则向字段添加填充以使其类似于C-struct
Copy - 制作dtype对象的新副本。 如果为false,则结果引用内置数据类型对象
例子1 (Example 1)
# using array-scalar type
import numpy as np
dt = np.dtype(np.int32)
print dt
输出如下 -
int32
例子2 (Example 2)
#int8, int16, int32, int64 can be replaced by equivalent string 'i1', 'i2','i4', etc.
import numpy as np
dt = np.dtype('i4')
print dt
输出如下 -
int32
例子3 (Example 3)
# using endian notation
import numpy as np
dt = np.dtype('>i4')
print dt
输出如下 -
>i4
以下示例显示了结构化数据类型的使用。 这里,将声明字段名称和相应的标量数据类型。
例子4 (Example 4)
# first create structured data type
import numpy as np
dt = np.dtype([('age',np.int8)])
print dt
输出如下 -
[('age', 'i1')]
例子5 (Example 5)
# now apply it to ndarray object
import numpy as np
dt = np.dtype([('age',np.int8)])
a = np.array([(10,),(20,),(30,)], dtype = dt)
print a
输出如下 -
[(10,) (20,) (30,)]
例6
# file name can be used to access content of age column
import numpy as np
dt = np.dtype([('age',np.int8)])
a = np.array([(10,),(20,),(30,)], dtype = dt)
print a['age']
输出如下 -
[10 20 30]
例7
以下示例定义了一个名为student的结构化数据类型,其中包含字符串字段“name”, integer field “age”和float field “marks”。 此dtype应用于ndarray对象。
import numpy as np
student = np.dtype([('name','S20'), ('age', 'i1'), ('marks', 'f4')])
print student
输出如下 -
[('name', 'S20'), ('age', 'i1'), ('marks', '<f4')])
例8
import numpy as np
student = np.dtype([('name','S20'), ('age', 'i1'), ('marks', 'f4')])
a = np.array([('abc', 21, 50),('xyz', 18, 75)], dtype = student)
print a
输出如下 -
[('abc', 21, 50.0), ('xyz', 18, 75.0)]
每个内置数据类型都有一个唯一标识它的字符代码。
'b' - 布尔值
'i' - (签名)整数
'u' - 无符号整数
'f' - 浮点数
'c' - 复杂浮点
'm' - timedelta
'M' - 日期时间
'O' - (Python)对象
'S', 'a' - (byte-)字符串
'U' - Unicode
'V' - 原始数据(无效)
NumPy - Array Attributes
在本章中,我们将讨论NumPy的各种数组属性。
ndarray.shape
此数组属性返回由数组维度组成的元组。 它也可以用于调整阵列的大小。
例子1 (Example 1)
import numpy as np
a = np.array([[1,2,3],[4,5,6]])
print a.shape
输出如下 -
(2, 3)
例子2 (Example 2)
# this resizes the ndarray
import numpy as np
a = np.array([[1,2,3],[4,5,6]])
a.shape = (3,2)
print a
输出如下 -
[[1, 2]
[3, 4]
[5, 6]]
例子3 (Example 3)
NumPy还提供了重塑函数来调整数组大小。
import numpy as np
a = np.array([[1,2,3],[4,5,6]])
b = a.reshape(3,2)
print b
输出如下 -
[[1, 2]
[3, 4]
[5, 6]]
ndarray.ndim
此数组属性返回数组维数。
例子1 (Example 1)
# an array of evenly spaced numbers
import numpy as np
a = np.arange(24)
print a
输出如下 -
[0 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23]
例子2 (Example 2)
# this is one dimensional array
import numpy as np
a = np.arange(24)
a.ndim
# now reshape it
b = a.reshape(2,4,3)
print b
# b is having three dimensions
输出如下 -
[[[ 0, 1, 2]
[ 3, 4, 5]
[ 6, 7, 8]
[ 9, 10, 11]]
[[12, 13, 14]
[15, 16, 17]
[18, 19, 20]
[21, 22, 23]]]
numpy.itemsize
此数组属性以字节为单位返回数组的每个元素的长度。
例子1 (Example 1)
# dtype of array is int8 (1 byte)
import numpy as np
x = np.array([1,2,3,4,5], dtype = np.int8)
print x.itemsize
输出如下 -
1
例子2 (Example 2)
# dtype of array is now float32 (4 bytes)
import numpy as np
x = np.array([1,2,3,4,5], dtype = np.float32)
print x.itemsize
输出如下 -
4
numpy.flags
ndarray对象具有以下属性。 其当前值由此函数返回。
Sr.No. | 属性和描述 |
---|---|
1 | C_CONTIGUOUS (C) 数据位于单个C风格的连续段中 |
2 | F_CONTIGUOUS (F) 数据位于Fortran风格的单个连续段中 |
3 | OWNDATA (O) 该数组拥有它使用的内存或从另一个对象借用它 |
4 | WRITEABLE (W) 可以写入数据区域。 将此设置为False将锁定数据,使其成为只读 |
5 | ALIGNED (A) 数据和所有元素都适合硬件对齐 |
6 | UPDATEIFCOPY (U) 此数组是其他一些数组的副本。 取消分配此数组时,将使用此数组的内容更新基本数组 |
例子 (Example)
以下示例显示了标志的当前值。
import numpy as np
x = np.array([1,2,3,4,5])
print x.flags
输出如下 -
C_CONTIGUOUS : True
F_CONTIGUOUS : True
OWNDATA : True
WRITEABLE : True
ALIGNED : True
UPDATEIFCOPY : False
NumPy - Array Creation Routines
可以通过以下任何数组创建例程或使用低级ndarray构造函数构造新的ndarray对象。
numpy.empty
它创建一个指定形状和dtype的未初始化数组。 它使用以下构造函数 -
numpy.empty(shape, dtype = float, order = 'C')
构造函数采用以下参数。
Sr.No. | 参数和描述 |
---|---|
1 | Shape int的int或元组中的空数组的形状 |
2 | Dtype 期望的输出数据类型。 可选的 |
3 | Order 对于C风格的行主阵列,'C',对于FORTRAN样式的列主阵列,'F' |
例子 (Example)
以下代码显示了一个空数组的示例。
import numpy as np
x = np.empty([3,2], dtype = int)
print x
输出如下 -
[[22649312 1701344351]
[1818321759 1885959276]
[16779776 156368896]]
Note - 数组中的元素显示随机值,因为它们未初始化。
numpy.zeros
返回指定大小的新数组,用零填充。
numpy.zeros(shape, dtype = float, order = 'C')
构造函数采用以下参数。
Sr.No. | 参数和描述 |
---|---|
1 | Shape int或int序列中空数组的形状 |
2 | Dtype 期望的输出数据类型。 可选的 |
3 | Order 对于C风格的行主阵列,'C',对于FORTRAN样式的列主阵列,'F' |
例子1 (Example 1)
# array of five zeros. Default dtype is float
import numpy as np
x = np.zeros(5)
print x
输出如下 -
[ 0. 0. 0. 0. 0.]
例子2 (Example 2)
import numpy as np
x = np.zeros((5,), dtype = np.int)
print x
现在,输出如下 -
[0 0 0 0 0]
例子3 (Example 3)
# custom type
import numpy as np
x = np.zeros((2,2), dtype = [('x', 'i4'), ('y', 'i4')])
print x
它应该产生以下输出 -
[[(0,0)(0,0)]
[(0,0)(0,0)]]
numpy.ones
返回指定大小和类型的新数组,用1填充。
numpy.ones(shape, dtype = None, order = 'C')
构造函数采用以下参数。
Sr.No. | 参数和描述 |
---|---|
1 | Shape int的int或元组中的空数组的形状 |
2 | Dtype 期望的输出数据类型。 可选的 |
3 | Order 对于C风格的行主阵列,'C',对于FORTRAN样式的列主阵列,'F' |
例子1 (Example 1)
# array of five ones. Default dtype is float
import numpy as np
x = np.ones(5)
print x
输出如下 -
[ 1. 1. 1. 1. 1.]
例子2 (Example 2)
import numpy as np
x = np.ones([2,2], dtype = int)
print x
现在,输出如下 -
[[1 1]
[1 1]]
NumPy - Array From Existing Data
在本章中,我们将讨论如何从现有数据创建数组。
numpy.asarray
此函数类似于numpy.array,除了它具有较少的参数。 此例程对于将Python序列转换为ndarray非常有用。
numpy.asarray(a, dtype = None, order = None)
构造函数采用以下参数。
Sr.No. | 参数和描述 |
---|---|
1 | a 以任何形式输入数据,例如列表,元组列表,元组,元组元组或列表元组 |
2 | dtype 默认情况下,输入数据的数据类型将应用于生成的ndarray |
3 | order C(行专业)或F(专业专业)。 C是默认的 |
以下示例显示了如何使用asarray函数。
例子1 (Example 1)
# convert list to ndarray
import numpy as np
x = [1,2,3]
a = np.asarray(x)
print a
其产出如下 -
[1 2 3]
例子2 (Example 2)
# dtype is set
import numpy as np
x = [1,2,3]
a = np.asarray(x, dtype = float)
print a
现在,输出如下 -
[ 1. 2. 3.]
例子3 (Example 3)
# ndarray from tuple
import numpy as np
x = (1,2,3)
a = np.asarray(x)
print a
它的输出是 -
[1 2 3]
例子4 (Example 4)
# ndarray from list of tuples
import numpy as np
x = [(1,2,3),(4,5)]
a = np.asarray(x)
print a
这里的输出如下 -
[(1, 2, 3) (4, 5)]
numpy.frombuffer
此函数将缓冲区解释为一维数组。 暴露缓冲区接口的任何对象都用作返回ndarray参数。
numpy.frombuffer(buffer, dtype = float, count = -1, offset = 0)
构造函数采用以下参数。
Sr.No. | 参数和描述 |
---|---|
1 | buffer 任何暴露缓冲区接口的对象 |
2 | dtype 返回的ndarray的数据类型。 默认为浮动 |
3 | count 要读取的项目数,默认值-1表示所有数据 |
4 | offset 从中读取的起始位置。 默认值为0 |
例子 (Example)
以下示例演示了frombuffer函数的frombuffer 。
import numpy as np
s = 'Hello World'
a = np.frombuffer(s, dtype = 'S1')
print a
这是它的输出 -
['H' 'e' 'l' 'l' 'o' ' ' 'W' 'o' 'r' 'l' 'd']
numpy.fromiter
此函数从任何可迭代对象构建ndarray对象。 此函数返回一个新的一维数组。
numpy.fromiter(iterable, dtype, count = -1)
这里,构造函数采用以下参数。
Sr.No. | 参数和描述 |
---|---|
1 | iterable 任何可迭代的对象 |
2 | dtype 结果数组的数据类型 |
3 | count 从迭代器中读取的项数。 默认值为-1表示要读取的所有数据 |
以下示例显示如何使用内置range()函数返回列表对象。 此列表的迭代器用于形成ndarray对象。
例子1 (Example 1)
# create list object using range function
import numpy as np
list = range(5)
print list
其输出如下 -
[0, 1, 2, 3, 4]
例子2 (Example 2)
# obtain iterator object from list
import numpy as np
list = range(5)
it = iter(list)
# use iterator to create ndarray
x = np.fromiter(it, dtype = float)
print x
现在,输出如下 -
[0. 1. 2. 3. 4.]
NumPy - Array From Numerical Ranges
在本章中,我们将了解如何从数值范围创建数组。
numpy.arange
此函数返回一个ndarray对象,该对象包含给定范围内的均匀间隔值。 功能的格式如下 -
numpy.arange(start, stop, step, dtype)
构造函数采用以下参数。
Sr.No. | 参数和描述 |
---|---|
1 | start 间隔的开始。 如果省略,则默认为0 |
2 | stop 间隔结束(不包括此数字) |
3 | step 值之间的间距,默认为1 |
4 | dtype 产生的ndarray的数据类型。 如果没有给出,则使用输入的数据类型 |
以下示例显示了如何使用此功能。
例子1 (Example 1)
import numpy as np
x = np.arange(5)
print x
其产出如下 -
[0 1 2 3 4]
例子2 (Example 2)
import numpy as np
# dtype set
x = np.arange(5, dtype = float)
print x
在这里,输出将是 -
[0. 1. 2. 3. 4.]
例子3 (Example 3)
# start and stop parameters set
import numpy as np
x = np.arange(10,20,2)
print x
其输出如下 -
[10 12 14 16 18]
numpy.linspace
此函数类似于arange()函数。 在此函数中,指定间隔之间的均匀间隔值,而不是步长。 该功能的用法如下 -
numpy.linspace(start, stop, num, endpoint, retstep, dtype)
构造函数采用以下参数。
Sr.No. | 参数和描述 |
---|---|
1 | start 序列的起始值 |
2 | stop 序列的结束值,如果endpoint设置为true,则包含在序列中 |
3 | num 要生成的均匀间隔样本的数量。 默认值为50 |
4 | endpoint 默认为True,因此停止值包含在序列中。 如果为false,则不包括在内 |
5 | retstep 如果为true,则返回样本并在连续数字之间切换 |
6 | dtype 输出数据类型ndarray |
以下示例演示了使用linspace函数。
例子1 (Example 1)
import numpy as np
x = np.linspace(10,20,5)
print x
它的输出是 -
[10. 12.5 15. 17.5 20.]
例子2 (Example 2)
# endpoint set to false
import numpy as np
x = np.linspace(10,20, 5, endpoint = False)
print x
输出将是 -
[10. 12. 14. 16. 18.]
例子3 (Example 3)
# find retstep value
import numpy as np
x = np.linspace(1,2,5, retstep = True)
print x
# retstep here is 0.25
现在,输出将是 -
(array([ 1. , 1.25, 1.5 , 1.75, 2. ]), 0.25)
numpy.logspace
此函数返回一个ndarray对象,该对象包含在对数刻度上均匀分布的数字。 开始和停止比例的终点是基数的索引,通常为10。
numpy.logspace(start, stop, num, endpoint, base, dtype)
以下参数确定logspace函数的输出。
Sr.No. | 参数和描述 |
---|---|
1 | start 序列的起点是基础开始 |
2 | stop 序列的最终值是基本停止 |
3 | num 范围之间的值的数量。 默认值为50 |
4 | endpoint 如果为true,则stop是范围中的最后一个值 |
5 | base 日志空间的基础,默认为10 |
6 | dtype 输出数组的数据类型。 如果没有给出,则取决于其他输入参数 |
以下示例将帮助您了解logspace功能。
例子1 (Example 1)
import numpy as np
# default base is 10
a = np.logspace(1.0, 2.0, num = 10)
print a
其产出如下 -
[ 10. 12.91549665 16.68100537 21.5443469 27.82559402
35.93813664 46.41588834 59.94842503 77.42636827 100. ]
例子2 (Example 2)
# set base of log space to 2
import numpy as np
a = np.logspace(1,10,num = 10, base = 2)
print a
现在,输出将是 -
[ 2. 4. 8. 16. 32. 64. 128. 256. 512. 1024.]
NumPy - Indexing & Slicing
可以通过索引或切片来访问和修改ndarray对象的内容,就像Python的内置容器对象一样。
如前所述,ndarray对象中的项遵循从零开始的索引。 有三种类型的索引方法可用 - field access, basic slicing和advanced indexing 。
基本切片是Python切片到n维的基本概念的扩展。 通过向内置slice函数提供start, stop和step参数来构造Python切片对象。 将此切片对象传递给数组以提取数组的一部分。
例子1 (Example 1)
import numpy as np
a = np.arange(10)
s = slice(2,7,2)
print a[s]
其输出如下 -
[2 4 6]
在上面的示例中, ndarray对象由arange()函数准备。 然后,分别用开始,停止和步骤值2,7和2定义切片对象。 当这个切片对象传递给ndarray时,它的一部分从索引2开始直到7,步长为2。
通过将冒号:(start:stop:step)分隔的切片参数直接赋予ndarray对象,也可以获得相同的结果。
例子2 (Example 2)
import numpy as np
a = np.arange(10)
b = a[2:7:2]
print b
在这里,我们将获得相同的输出 -
[2 4 6]
如果只放置一个参数,则将返回与索引对应的单个项目。 如果在其前面插入:,则将提取该索引以后的所有项目。 如果使用两个参数(在它们之间使用:),则会对具有默认第一步的两个索引(不包括停止索引)之间的项进行切片。
例子3 (Example 3)
# slice single item
import numpy as np
a = np.arange(10)
b = a[5]
print b
其输出如下 -
5
例子4 (Example 4)
# slice items starting from index
import numpy as np
a = np.arange(10)
print a[2:]
现在,输出将是 -
[2 3 4 5 6 7 8 9]
例5
# slice items between indexes
import numpy as np
a = np.arange(10)
print a[2:5]
在这里,输出将是 -
[2 3 4]
以上描述也适用于多维ndarray 。
例6
import numpy as np
a = np.array([[1,2,3],[3,4,5],[4,5,6]])
print a
# slice items starting from index
print 'Now we will slice the array from the index a[1:]'
print a[1:]
输出如下 -
[[1 2 3]
[3 4 5]
[4 5 6]]
Now we will slice the array from the index a[1:]
[[3 4 5]
[4 5 6]]
切片还可以包括省略号(...),以生成与数组维度长度相同的选择元组。 如果在行位置使用省略号,它将返回包含行中项目的ndarray。
例7
# array to begin with
import numpy as np
a = np.array([[1,2,3],[3,4,5],[4,5,6]])
print 'Our array is:'
print a
print '\n'
# this returns array of items in the second column
print 'The items in the second column are:'
print a[...,1]
print '\n'
# Now we will slice all items from the second row
print 'The items in the second row are:'
print a[1,...]
print '\n'
# Now we will slice all items from column 1 onwards
print 'The items column 1 onwards are:'
print a[...,1:]
该计划的产出如下 -
Our array is:
[[1 2 3]
[3 4 5]
[4 5 6]]
The items in the second column are:
[2 4 5]
The items in the second row are:
[3 4 5]
The items column 1 onwards are:
[[2 3]
[4 5]
[5 6]]
NumPy - Advanced Indexing
可以从ndarray中选择非元组序列,整数或布尔数据类型的ndarray对象,或者至少有一个项目是序列对象的元组。 高级索引始终返回数据的副本。 与此相反,切片仅呈现视图。
高级索引有两种类型 - Integer和Boolean 。
整数索引
此机制有助于根据其N维索引选择数组中的任意项。 每个整数数组表示该维度的索引数。 当索引由与目标ndarray的维度一样多的整数数组组成时,它变得简单明了。
在以下示例中,选择了ndarray对象的每一行中指定列的一个元素。 因此,行索引包含所有行号,列索引指定要选择的元素。
例子1 (Example 1)
import numpy as np
x = np.array([[1, 2], [3, 4], [5, 6]])
y = x[[0,1,2], [0,1,0]]
print y
其产出如下 -
[1 4 5]
该选择包括来自第一阵列的(0,0),(1,1)和(2,0)处的元素。
在以下示例中,选择放置在4X3阵列角落的元素。 选择的行索引是[0,0]和[3,3],而列索引是[0,2]和[0,2]。
例子2 (Example 2)
import numpy as np
x = np.array([[ 0, 1, 2],[ 3, 4, 5],[ 6, 7, 8],[ 9, 10, 11]])
print 'Our array is:'
print x
print '\n'
rows = np.array([[0,0],[3,3]])
cols = np.array([[0,2],[0,2]])
y = x[rows,cols]
print 'The corner elements of this array are:'
print y
该计划的产出如下 -
Our array is:
[[ 0 1 2]
[ 3 4 5]
[ 6 7 8]
[ 9 10 11]]
The corner elements of this array are:
[[ 0 2]
[ 9 11]]
结果选择是包含角元素的ndarray对象。
可以通过使用带有索引数组的一个切片(:)或省略号(...)来组合高级和基本索引。 以下示例使用slice for row和advanced index for column。 当切片用于两者时,结果是相同的。 但高级索引会导致复制,并且可能具有不同的内存布局。
例子3 (Example 3)
import numpy as np
x = np.array([[ 0, 1, 2],[ 3, 4, 5],[ 6, 7, 8],[ 9, 10, 11]])
print 'Our array is:'
print x
print '\n'
# slicing
z = x[1:4,1:3]
print 'After slicing, our array becomes:'
print z
print '\n'
# using advanced index for column
y = x[1:4,[1,2]]
print 'Slicing using advanced index for column:'
print y
该计划的产出如下 -
Our array is:
[[ 0 1 2]
[ 3 4 5]
[ 6 7 8]
[ 9 10 11]]
After slicing, our array becomes:
[[ 4 5]
[ 7 8]
[10 11]]
Slicing using advanced index for column:
[[ 4 5]
[ 7 8]
[10 11]]
布尔数组索引
当结果对象是布尔运算的结果(例如比较运算符)时,将使用此类高级索引。
例子1 (Example 1)
在此示例中,作为布尔索引的结果,返回大于5的项。
import numpy as np
x = np.array([[ 0, 1, 2],[ 3, 4, 5],[ 6, 7, 8],[ 9, 10, 11]])
print 'Our array is:'
print x
print '\n'
# Now we will print the items greater than 5
print 'The items greater than 5 are:'
print x[x > 5]
该计划的产出将是 -
Our array is:
[[ 0 1 2]
[ 3 4 5]
[ 6 7 8]
[ 9 10 11]]
The items greater than 5 are:
[ 6 7 8 9 10 11]
例子2 (Example 2)
在此示例中,使用〜(补码运算符)省略了NaN(非数字)元素。
import numpy as np
a = np.array([np.nan, 1,2,np.nan,3,4,5])
print a[~np.isnan(a)]
它的输出是 -
[ 1. 2. 3. 4. 5.]
例子3 (Example 3)
以下示例显示如何从数组中过滤掉非复杂元素。
import numpy as np
a = np.array([1, 2+6j, 5, 3.5+5j])
print a[np.iscomplex(a)]
这里的输出如下 -
[2.0+6.j 3.5+5.j]
NumPy - Broadcasting
术语broadcasting是指NumPy在算术运算期间处理不同形状的阵列的能力。 对数组的算术运算通常在相应的元素上完成。 如果两个阵列具有完全相同的形状,则可以平滑地执行这些操作。
例子1 (Example 1)
import numpy as np
a = np.array([1,2,3,4])
b = np.array([10,20,30,40])
c = a * b
print c
其输出如下 -
[10 40 90 160]
如果两个数组的维度不同,则无法进行元素到元素的操作。 但是,由于广播能力,在NumPy中仍然可以对非相似形状的阵列进行操作。 较小的阵列被broadcast到较大阵列的大小,以便它们具有兼容的形状。
如果满足以下规则,则可以进行广播 -
ndim小于另一个的数组在其形状前面加上'1'。
输出形状的每个维度的大小是该维度中输入大小的最大值。
如果输入在特定维度中的大小与输出大小匹配或者其值恰好为1,则可以在计算中使用该输入。
如果输入的维度大小为1,则该维度中的第一个数据条目将用于该维度上的所有计算。
如果上述规则产生有效结果且下列之一为真,则称一组数组是可broadcastable的 -
阵列具有完全相同的形状。
数组具有相同数量的维度,每个维度的长度可以是常用长度或1。
具有太小尺寸的阵列可以使其形状预先具有长度为1的尺寸,使得上述特性成立。
以下程序显示了广播的示例。
例子2 (Example 2)
import numpy as np
a = np.array([[0.0,0.0,0.0],[10.0,10.0,10.0],[20.0,20.0,20.0],[30.0,30.0,30.0]])
b = np.array([1.0,2.0,3.0])
print 'First array:'
print a
print '\n'
print 'Second array:'
print b
print '\n'
print 'First Array + Second Array'
print a + b
该计划的产出如下 -
First array:
[[ 0. 0. 0.]
[ 10. 10. 10.]
[ 20. 20. 20.]
[ 30. 30. 30.]]
Second array:
[ 1. 2. 3.]
First Array + Second Array
[[ 1. 2. 3.]
[ 11. 12. 13.]
[ 21. 22. 23.]
[ 31. 32. 33.]]
下图演示了如何广播数组b以与a兼容。
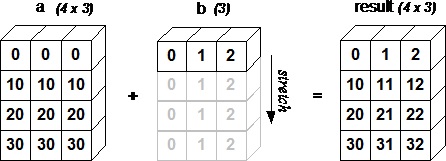
NumPy - Iterating Over Array
NumPy包中包含一个迭代器对象numpy.nditer 。 它是一个有效的多维迭代器对象,使用它可以迭代数组。 使用Python的标准Iterator接口访问数组的每个元素。
让我们使用nditer ()函数创建一个3X4数组,并使用nditer迭代它。
例子1 (Example 1)
import numpy as np
a = np.arange(0,60,5)
a = a.reshape(3,4)
print 'Original array is:'
print a
print '\n'
print 'Modified array is:'
for x in np.nditer(a):
print x,
该计划的产出如下 -
Original array is:
[[ 0 5 10 15]
[20 25 30 35]
[40 45 50 55]]
Modified array is:
0 5 10 15 20 25 30 35 40 45 50 55
例子2 (Example 2)
选择迭代的顺序以匹配阵列的存储器布局,而不考虑特定的排序。 通过迭代上述数组的转置可以看出这一点。
import numpy as np
a = np.arange(0,60,5)
a = a.reshape(3,4)
print 'Original array is:'
print a
print '\n'
print 'Transpose of the original array is:'
b = a.T
print b
print '\n'
print 'Modified array is:'
for x in np.nditer(b):
print x,
上述计划的输出如下 -
Original array is:
[[ 0 5 10 15]
[20 25 30 35]
[40 45 50 55]]
Transpose of the original array is:
[[ 0 20 40]
[ 5 25 45]
[10 30 50]
[15 35 55]]
Modified array is:
0 5 10 15 20 25 30 35 40 45 50 55
迭代顺序
如果使用F样式的顺序存储相同的元素,迭代器会选择迭代数组的更有效方法。
例子1 (Example 1)
import numpy as np
a = np.arange(0,60,5)
a = a.reshape(3,4)
print 'Original array is:'
print a
print '\n'
print 'Transpose of the original array is:'
b = a.T
print b
print '\n'
print 'Sorted in C-style order:'
c = b.copy(order='C')
print c
for x in np.nditer(c):
print x,
print '\n'
print 'Sorted in F-style order:'
c = b.copy(order='F')
print c
for x in np.nditer(c):
print x,
其产出如下 -
Original array is:
[[ 0 5 10 15]
[20 25 30 35]
[40 45 50 55]]
Transpose of the original array is:
[[ 0 20 40]
[ 5 25 45]
[10 30 50]
[15 35 55]]
Sorted in C-style order:
[[ 0 20 40]
[ 5 25 45]
[10 30 50]
[15 35 55]]
0 20 40 5 25 45 10 30 50 15 35 55
Sorted in F-style order:
[[ 0 20 40]
[ 5 25 45]
[10 30 50]
[15 35 55]]
0 5 10 15 20 25 30 35 40 45 50 55
例子2 (Example 2)
通过明确提及它,可以强制nditer对象使用特定的顺序。
import numpy as np
a = np.arange(0,60,5)
a = a.reshape(3,4)
print 'Original array is:'
print a
print '\n'
print 'Sorted in C-style order:'
for x in np.nditer(a, order = 'C'):
print x,
print '\n'
print 'Sorted in F-style order:'
for x in np.nditer(a, order = 'F'):
print x,
它的输出是 -
Original array is:
[[ 0 5 10 15]
[20 25 30 35]
[40 45 50 55]]
Sorted in C-style order:
0 5 10 15 20 25 30 35 40 45 50 55
Sorted in F-style order:
0 20 40 5 25 45 10 30 50 15 35 55
修改数组值
nditer对象有另一个名为op_flags可选参数。 其默认值为只读,但可以设置为读写或只写模式。 这将允许使用此迭代器修改数组元素。
例子 (Example)
import numpy as np
a = np.arange(0,60,5)
a = a.reshape(3,4)
print 'Original array is:'
print a
print '\n'
for x in np.nditer(a, op_flags = ['readwrite']):
x[...] = 2*x
print 'Modified array is:'
print a
其输出如下 -
Original array is:
[[ 0 5 10 15]
[20 25 30 35]
[40 45 50 55]]
Modified array is:
[[ 0 10 20 30]
[ 40 50 60 70]
[ 80 90 100 110]]
外部循环
nditer类构造函数有一个'flags'参数,它可以采用以下值 -
Sr.No. | 参数和描述 |
---|---|
1 | c_index 可以跟踪C_order索引 |
2 | f_index 追踪Fortran_order索引 |
3 | multi-index 可以跟踪每次迭代一次的索引类型 |
4 | external_loop 导致给定的值是具有多个值而不是零维数组的一维数组 |
例子 (Example)
在以下示例中,迭代器遍历与每列对应的一维数组。
import numpy as np
a = np.arange(0,60,5)
a = a.reshape(3,4)
print 'Original array is:'
print a
print '\n'
print 'Modified array is:'
for x in np.nditer(a, flags = ['external_loop'], order = 'F'):
print x,
输出如下 -
Original array is:
[[ 0 5 10 15]
[20 25 30 35]
[40 45 50 55]]
Modified array is:
[ 0 20 40] [ 5 25 45] [10 30 50] [15 35 55]
广播迭代
如果两个数组是可broadcastable ,则组合的nditer对象能够同时迭代它们。 假设数组a维度为3X4,并且存在另一个维度为1X4的数组b ,则使用以下类型的迭代器(数组b广播为b大小)。
例子 (Example)
import numpy as np
a = np.arange(0,60,5)
a = a.reshape(3,4)
print 'First array is:'
print a
print '\n'
print 'Second array is:'
b = np.array([1, 2, 3, 4], dtype = int)
print b
print '\n'
print 'Modified array is:'
for x,y in np.nditer([a,b]):
print "%d:%d" % (x,y),
其产出如下 -
First array is:
[[ 0 5 10 15]
[20 25 30 35]
[40 45 50 55]]
Second array is:
[1 2 3 4]
Modified array is:
0:1 5:2 10:3 15:4 20:1 25:2 30:3 35:4 40:1 45:2 50:3 55:4
NumPy - Array Manipulation
NumPy包中有几个例程可用于操作ndarray对象中的元素。 它们可分为以下类型 -
改变形状
Sr.No. | 形状和描述 |
---|---|
1 | reshape 为数组提供新形状而不更改其数据 |
2 | flat 数组上的一维迭代器 |
3 | flatten 返回折叠为一维的数组的副本 |
4 | ravel 返回连续的展平数组 |
转置操作
Sr.No. | 操作和说明 |
---|---|
1 | transpose 置换数组的维度 |
2 | ndarray.T 与self.transpose()相同 |
3 | rollaxis 向后滚动指定的轴 |
4 | swapaxes 交换阵列的两个轴 |
改变尺寸
Sr.No. | 尺寸和描述 |
---|---|
1 | broadcast 生成模仿广播的对象 |
2 | broadcast_to 将数组广播为新形状 |
3 | expand_dims 扩展数组的形状 |
4 | squeeze 从数组的形状中删除一维条目 |
加入阵列
Sr.No. | 数组和描述 |
---|---|
1 | concatenate 沿现有轴连接一系列数组 |
2 | stack 沿新轴连接一系列数组 |
3 | hstack 按顺序堆叠数组(列式) |
4 | vstack 垂直堆叠数组(行方式) |
分裂数组
Sr.No. | 数组和描述 |
---|---|
1 | split 将数组拆分为多个子数组 |
2 | hsplit 将数组水平拆分为多个子数组(逐列) |
3 | vsplit 将数组垂直拆分为多个子数组(行方式) |
添加/删除元素
Sr.No. | 元素和描述 |
---|---|
1 | resize 返回具有指定形状的新数组 |
2 | append 将值追加到数组的末尾 |
3 | insert 在给定索引之前沿给定轴插入值 |
4 | delete 返回一个新数组,其子轴数组沿轴被删除 |
5 | unique 查找数组的唯一元素 |
NumPy - Binary Operators
以下是NumPy包中可用的按位运算功能。
Sr.No. | 操作和说明 |
---|---|
1 | bitwise_and 计算数组元素的按位AND运算 |
2 | bitwise_or 计算数组元素的按位OR运算 |
3 | invert 按位计算NOT |
4 | left_shift 将二进制表示的位向左移位 |
5 | right_shift 将二进制表示的位向右移位 |
NumPy - 字符串函数
以下函数用于对dtype numpy.string_或numpy.unicode_的数组执行矢量化字符串操作。 它们基于Python内置库中的标准字符串函数。
Sr.No. | 功能说明 |
---|---|
1 | add() 返回两个str或Unicode数组的逐元素字符串连接 |
2 | multiply() 以元素方式返回具有多个串联的字符串 |
3 | center() 返回给定字符串的副本,其中元素以指定长度的字符串为中心 |
4 | capitalize() 返回只有第一个字符大写的字符串的副本 |
5 | title() 返回字符串或unicode的按元素标题的版本 |
6 | lower() 返回一个数组,其元素转换为小写 |
7 | upper() 返回一个数组,其元素转换为大写 |
8 | split() 使用separatordelimiter返回字符串中单词的列表 |
9 | splitlines() 返回元素中的行列表,在行边界处断开 |
10 | strip() 返回删除了前导和尾随字符的副本 |
11 | join() 返回一个字符串,该字符串是序列中字符串的串联 |
12 | replace() 返回字符串的副本,其中所有出现的子字符串都被新字符串替换 |
13 | decode() 以元素方式调用str.decode |
14 | encode() 以元素方式调用str.encode |
这些函数在字符数组类(numpy.char)中定义。 较旧的Numarray包中包含chararray类。 numpy.char类中的上述函数在执行向量化字符串操作时很有用。
NumPy - 数学函数 (NumPy - Mathematical Functions)
可以理解的是,NumPy包含大量的各种数学运算。 NumPy提供标准的三角函数,算术运算函数,复数等处理。
三角函数 (Trigonometric Functions)
NumPy具有标准的三角函数,它以弧度为单位返回给定角度的三角比。
Example
import numpy as np
a = np.array([0,30,45,60,90])
print 'Sine of different angles:'
# Convert to radians by multiplying with pi/180
print np.sin(a*np.pi/180)
print '\n'
print 'Cosine values for angles in array:'
print np.cos(a*np.pi/180)
print '\n'
print 'Tangent values for given angles:'
print np.tan(a*np.pi/180)
这是它的输出 -
Sine of different angles:
[ 0. 0.5 0.70710678 0.8660254 1. ]
Cosine values for angles in array:
[ 1.00000000e+00 8.66025404e-01 7.07106781e-01 5.00000000e-01
6.12323400e-17]
Tangent values for given angles:
[ 0.00000000e+00 5.77350269e-01 1.00000000e+00 1.73205081e+00
1.63312394e+16]
arcsin, arcos,和arctan函数返回给定角度的sin,cos和tan的三角逆。 通过将弧度转换为度数, numpy.degrees() function可以验证这些函数的结果。
Example
import numpy as np
a = np.array([0,30,45,60,90])
print 'Array containing sine values:'
sin = np.sin(a*np.pi/180)
print sin
print '\n'
print 'Compute sine inverse of angles. Returned values are in radians.'
inv = np.arcsin(sin)
print inv
print '\n'
print 'Check result by converting to degrees:'
print np.degrees(inv)
print '\n'
print 'arccos and arctan functions behave similarly:'
cos = np.cos(a*np.pi/180)
print cos
print '\n'
print 'Inverse of cos:'
inv = np.arccos(cos)
print inv
print '\n'
print 'In degrees:'
print np.degrees(inv)
print '\n'
print 'Tan function:'
tan = np.tan(a*np.pi/180)
print tan
print '\n'
print 'Inverse of tan:'
inv = np.arctan(tan)
print inv
print '\n'
print 'In degrees:'
print np.degrees(inv)
其输出如下 -
Array containing sine values:
[ 0. 0.5 0.70710678 0.8660254 1. ]
Compute sine inverse of angles. Returned values are in radians.
[ 0. 0.52359878 0.78539816 1.04719755 1.57079633]
Check result by converting to degrees:
[ 0. 30. 45. 60. 90.]
arccos and arctan functions behave similarly:
[ 1.00000000e+00 8.66025404e-01 7.07106781e-01 5.00000000e-01
6.12323400e-17]
Inverse of cos:
[ 0. 0.52359878 0.78539816 1.04719755 1.57079633]
In degrees:
[ 0. 30. 45. 60. 90.]
Tan function:
[ 0.00000000e+00 5.77350269e-01 1.00000000e+00 1.73205081e+00
1.63312394e+16]
Inverse of tan:
[ 0. 0.52359878 0.78539816 1.04719755 1.57079633]
In degrees:
[ 0. 30. 45. 60. 90.]
舍入功能
numpy.around()
这是一个函数,它返回舍入到所需精度的值。 该函数采用以下参数。
numpy.around(a,decimals)
Where,
Sr.No. | 参数和描述 |
---|---|
1 | a 输入数据 |
2 | decimals 要舍入的小数位数。 默认值为0.如果为负,则将整数舍入到小数点左侧的位置 |
Example
import numpy as np
a = np.array([1.0,5.55, 123, 0.567, 25.532])
print 'Original array:'
print a
print '\n'
print 'After rounding:'
print np.around(a)
print np.around(a, decimals = 1)
print np.around(a, decimals = -1)
它产生以下输出 -
Original array:
[ 1. 5.55 123. 0.567 25.532]
After rounding:
[ 1. 6. 123. 1. 26. ]
[ 1. 5.6 123. 0.6 25.5]
[ 0. 10. 120. 0. 30. ]
numpy.floor()
此函数返回不大于输入参数的最大整数。 scalar x的最大值是最大的integer i ,因此i 《= x 。 请注意,在Python中,地板总是从0开始舍入。
Example
import numpy as np
a = np.array([-1.7, 1.5, -0.2, 0.6, 10])
print 'The given array:'
print a
print '\n'
print 'The modified array:'
print np.floor(a)
它产生以下输出 -
The given array:
[ -1.7 1.5 -0.2 0.6 10. ]
The modified array:
[ -2. 1. -1. 0. 10.]
numpy.ceil()
ceil()函数返回输入值的上限,即scalar x的ceil是最小的integer i ,使得i 》= x.
Example
import numpy as np
a = np.array([-1.7, 1.5, -0.2, 0.6, 10])
print 'The given array:'
print a
print '\n'
print 'The modified array:'
print np.ceil(a)
它将产生以下输出 -
The given array:
[ -1.7 1.5 -0.2 0.6 10. ]
The modified array:
[ -1. 2. -0. 1. 10.]
NumPy - Arithmetic Operations
用于执行算术运算的输入数组(如add(),subtract(),multiply()和divide())必须具有相同的形状或者应符合数组广播规则。
例子 (Example)
import numpy as np
a = np.arange(9, dtype = np.float_).reshape(3,3)
print 'First array:'
print a
print '\n'
print 'Second array:'
b = np.array([10,10,10])
print b
print '\n'
print 'Add the two arrays:'
print np.add(a,b)
print '\n'
print 'Subtract the two arrays:'
print np.subtract(a,b)
print '\n'
print 'Multiply the two arrays:'
print np.multiply(a,b)
print '\n'
print 'Divide the two arrays:'
print np.divide(a,b)
它将产生以下输出 -
First array:
[[ 0. 1. 2.]
[ 3. 4. 5.]
[ 6. 7. 8.]]
Second array:
[10 10 10]
Add the two arrays:
[[ 10. 11. 12.]
[ 13. 14. 15.]
[ 16. 17. 18.]]
Subtract the two arrays:
[[-10. -9. -8.]
[ -7. -6. -5.]
[ -4. -3. -2.]]
Multiply the two arrays:
[[ 0. 10. 20.]
[ 30. 40. 50.]
[ 60. 70. 80.]]
Divide the two arrays:
[[ 0. 0.1 0.2]
[ 0.3 0.4 0.5]
[ 0.6 0.7 0.8]]
现在让我们讨论一下NumPy中可用的其他一些重要的算术函数。
numpy.reciprocal()
此函数以元素方式返回参数的倒数。 对于绝对值大于1的元素,结果始终为0,因为Python处理整数除法的方式。 对于整数0,发出溢出警告。
例子 (Example)
import numpy as np
a = np.array([0.25, 1.33, 1, 0, 100])
print 'Our array is:'
print a
print '\n'
print 'After applying reciprocal function:'
print np.reciprocal(a)
print '\n'
b = np.array([100], dtype = int)
print 'The second array is:'
print b
print '\n'
print 'After applying reciprocal function:'
print np.reciprocal(b)
它将产生以下输出 -
Our array is:
[ 0.25 1.33 1. 0. 100. ]
After applying reciprocal function:
main.py:9: RuntimeWarning: divide by zero encountered in reciprocal
print np.reciprocal(a)
[ 4. 0.7518797 1. inf 0.01 ]
The second array is:
[100]
After applying reciprocal function:
[0]
numpy.power()
此函数将第一个输入数组中的元素视为基数,并将其提升为第二个输入数组中相应元素的幂。
import numpy as np
a = np.array([10,100,1000])
print 'Our array is:'
print a
print '\n'
print 'Applying power function:'
print np.power(a,2)
print '\n'
print 'Second array:'
b = np.array([1,2,3])
print b
print '\n'
print 'Applying power function again:'
print np.power(a,b)
它将产生以下输出 -
Our array is:
[ 10 100 1000]
Applying power function:
[ 100 10000 1000000]
Second array:
[1 2 3]
Applying power function again:
[ 10 10000 1000000000]
numpy.mod()
此函数返回输入数组中相应元素的剩余部分。 函数numpy.remainder()也产生相同的结果。
import numpy as np
a = np.array([10,20,30])
b = np.array([3,5,7])
print 'First array:'
print a
print '\n'
print 'Second array:'
print b
print '\n'
print 'Applying mod() function:'
print np.mod(a,b)
print '\n'
print 'Applying remainder() function:'
print np.remainder(a,b)
它将产生以下输出 -
First array:
[10 20 30]
Second array:
[3 5 7]
Applying mod() function:
[1 0 2]
Applying remainder() function:
[1 0 2]
以下函数用于对具有复数的数组执行操作。
numpy.real() - 返回复杂数据类型参数的实部。
numpy.imag() - 返回复杂数据类型参数的虚部。
numpy.conj() - 返回复共轭,它是通过改变虚部的符号得到的。
numpy.angle() - 返回复杂参数的角度。 该函数具有度参数。 如果为true,则返回度数中的角度,否则角度为弧度。
import numpy as np
a = np.array([-5.6j, 0.2j, 11. , 1+1j])
print 'Our array is:'
print a
print '\n'
print 'Applying real() function:'
print np.real(a)
print '\n'
print 'Applying imag() function:'
print np.imag(a)
print '\n'
print 'Applying conj() function:'
print np.conj(a)
print '\n'
print 'Applying angle() function:'
print np.angle(a)
print '\n'
print 'Applying angle() function again (result in degrees)'
print np.angle(a, deg = True)
它将产生以下输出 -
Our array is:
[ 0.-5.6j 0.+0.2j 11.+0.j 1.+1.j ]
Applying real() function:
[ 0. 0. 11. 1.]
Applying imag() function:
[-5.6 0.2 0. 1. ]
Applying conj() function:
[ 0.+5.6j 0.-0.2j 11.-0.j 1.-1.j ]
Applying angle() function:
[-1.57079633 1.57079633 0. 0.78539816]
Applying angle() function again (result in degrees)
[-90. 90. 0. 45.]
NumPy - 统计功能 (NumPy - Statistical Functions)
NumPy具有相当多的有用统计函数,用于从阵列中的给定元素中查找最小值,最大值,百分位数标准差和方差等。 功能说明如下 -
numpy.amin() and numpy.amax()
这些函数沿指定轴返回给定数组中元素的最小值和最大值。
例子 (Example)
import numpy as np
a = np.array([[3,7,5],[8,4,3],[2,4,9]])
print 'Our array is:'
print a
print '\n'
print 'Applying amin() function:'
print np.amin(a,1)
print '\n'
print 'Applying amin() function again:'
print np.amin(a,0)
print '\n'
print 'Applying amax() function:'
print np.amax(a)
print '\n'
print 'Applying amax() function again:'
print np.amax(a, axis = 0)
它将产生以下输出 -
Our array is:
[[3 7 5]
[8 4 3]
[2 4 9]]
Applying amin() function:
[3 3 2]
Applying amin() function again:
[2 4 3]
Applying amax() function:
9
Applying amax() function again:
[8 7 9]
numpy.ptp()
numpy.ptp()函数返回沿轴的值范围(最大值 - 最小值)。
import numpy as np
a = np.array([[3,7,5],[8,4,3],[2,4,9]])
print 'Our array is:'
print a
print '\n'
print 'Applying ptp() function:'
print np.ptp(a)
print '\n'
print 'Applying ptp() function along axis 1:'
print np.ptp(a, axis = 1)
print '\n'
print 'Applying ptp() function along axis 0:'
print np.ptp(a, axis = 0)
它将产生以下输出 -
Our array is:
[[3 7 5]
[8 4 3]
[2 4 9]]
Applying ptp() function:
7
Applying ptp() function along axis 1:
[4 5 7]
Applying ptp() function along axis 0:
[6 3 6]
numpy.percentile()
百分位数(或百分位数)是统计中使用的度量,指示一组观察中给定百分比的观察值下降的值。 函数numpy.percentile()接受以下参数。
numpy.percentile(a, q, axis)
Where,
Sr.No. | 论点和描述 |
---|---|
1 | a 输入数组 |
2 | q 计算的百分位数必须在0到100之间 |
3 | axis 用于计算百分位数的轴 |
例子 (Example)
import numpy as np
a = np.array([[30,40,70],[80,20,10],[50,90,60]])
print 'Our array is:'
print a
print '\n'
print 'Applying percentile() function:'
print np.percentile(a,50)
print '\n'
print 'Applying percentile() function along axis 1:'
print np.percentile(a,50, axis = 1)
print '\n'
print 'Applying percentile() function along axis 0:'
print np.percentile(a,50, axis = 0)
它将产生以下输出 -
Our array is:
[[30 40 70]
[80 20 10]
[50 90 60]]
Applying percentile() function:
50.0
Applying percentile() function along axis 1:
[ 40. 20. 60.]
Applying percentile() function along axis 0:
[ 50. 40. 60.]
numpy.median()
Median定义为将数据样本的较高一半与下半部分分开的值。 使用numpy.median()函数,如以下程序所示。
例子 (Example)
import numpy as np
a = np.array([[30,65,70],[80,95,10],[50,90,60]])
print 'Our array is:'
print a
print '\n'
print 'Applying median() function:'
print np.median(a)
print '\n'
print 'Applying median() function along axis 0:'
print np.median(a, axis = 0)
print '\n'
print 'Applying median() function along axis 1:'
print np.median(a, axis = 1)
它将产生以下输出 -
Our array is:
[[30 65 70]
[80 95 10]
[50 90 60]]
Applying median() function:
65.0
Applying median() function along axis 0:
[ 50. 90. 60.]
Applying median() function along axis 1:
[ 65. 80. 60.]
numpy.mean()
算术平均值是沿轴的元素之和除以元素的数量。 numpy.mean()函数返回数组中元素的算术平均值。 如果提到轴,则沿着它计算。
例子 (Example)
import numpy as np
a = np.array([[1,2,3],[3,4,5],[4,5,6]])
print 'Our array is:'
print a
print '\n'
print 'Applying mean() function:'
print np.mean(a)
print '\n'
print 'Applying mean() function along axis 0:'
print np.mean(a, axis = 0)
print '\n'
print 'Applying mean() function along axis 1:'
print np.mean(a, axis = 1)
它将产生以下输出 -
Our array is:
[[1 2 3]
[3 4 5]
[4 5 6]]
Applying mean() function:
3.66666666667
Applying mean() function along axis 0:
[ 2.66666667 3.66666667 4.66666667]
Applying mean() function along axis 1:
[ 2. 4. 5.]
numpy.average()
加权平均值是由每个分量乘以反映其重要性的因子得到的平均值。 numpy.average()函数根据另一个数组中给出的各自权重计算数组中元素的加权平均值。 该函数可以具有轴参数。 如果未指定轴,则数组将展平。
考虑阵列[1,2,3,4]和相应的权重[4,3,2,1],通过将相应元素的乘积相加并将总和除以权重之和来计算加权平均值。
加权平均值=(1 * 4 + 2 * 3 + 3 * 2 + 4 * 1)/(4 + 3 + 2 + 1)
例子 (Example)
import numpy as np
a = np.array([1,2,3,4])
print 'Our array is:'
print a
print '\n'
print 'Applying average() function:'
print np.average(a)
print '\n'
# this is same as mean when weight is not specified
wts = np.array([4,3,2,1])
print 'Applying average() function again:'
print np.average(a,weights = wts)
print '\n'
# Returns the sum of weights, if the returned parameter is set to True.
print 'Sum of weights'
print np.average([1,2,3, 4],weights = [4,3,2,1], returned = True)
它将产生以下输出 -
Our array is:
[1 2 3 4]
Applying average() function:
2.5
Applying average() function again:
2.0
Sum of weights
(2.0, 10.0)
在多维数组中,可以指定用于计算的轴。
例子 (Example)
import numpy as np
a = np.arange(6).reshape(3,2)
print 'Our array is:'
print a
print '\n'
print 'Modified array:'
wt = np.array([3,5])
print np.average(a, axis = 1, weights = wt)
print '\n'
print 'Modified array:'
print np.average(a, axis = 1, weights = wt, returned = True)
它将产生以下输出 -
Our array is:
[[0 1]
[2 3]
[4 5]]
Modified array:
[ 0.625 2.625 4.625]
Modified array:
(array([ 0.625, 2.625, 4.625]), array([ 8., 8., 8.]))
标准偏差
标准偏差是平均偏差平均值的平方根。 标准差的公式如下 -
std = sqrt(mean(abs(x - x.mean())**2))
如果数组是[1,2,3,4],那么它的平均值是2.5。 因此,平方偏差为[2.25,0.25,0.25,2.25],其平均值的平方根除以4,即sqrt(5/4)为1.1180339887498949。
例子 (Example)
import numpy as np
print np.std([1,2,3,4])
它将产生以下输出 -
1.1180339887498949
Variance
方差是平方偏差的平均值,即mean(abs(x - x.mean())**2) 。 换句话说,标准差是方差的平方根。
例子 (Example)
import numpy as np
print np.var([1,2,3,4])
它将产生以下输出 -
1.25
NumPy - Sort, Search & Counting Functions
NumPy中提供了各种与排序相关的功能。 这些排序函数实现了不同的排序算法,每个排序算法的特点是执行速度,最差情况性能,所需的工作空间和算法的稳定性。 下表显示了三种排序算法的比较。
类 | 速度 | 最糟糕的情况 | 工作空间 | 稳定 |
---|---|---|---|---|
‘quicksort’ | 1 | O(n^2) | 0 | no |
‘mergesort’ | 2 | O(n*log(n)) | ~n/2 | yes |
‘heapsort’ | 3 | O(n*log(n)) | 0 | no |
numpy.sort()
sort()函数返回输入数组的排序副本。 它有以下参数 -
numpy.sort(a, axis, kind, order)
Where,
Sr.No. | 参数和描述 |
---|---|
1 | a 要排序的数组 |
2 | axis 要对数组进行排序的轴。 如果不是,则数组被展平,在最后一个轴上排序 |
3 | kind 默认是快速排序 |
4 | order 如果数组包含字段,则要排序的字段的顺序 |
例子 (Example)
import numpy as np
a = np.array([[3,7],[9,1]])
print 'Our array is:'
print a
print '\n'
print 'Applying sort() function:'
print np.sort(a)
print '\n'
print 'Sort along axis 0:'
print np.sort(a, axis = 0)
print '\n'
# Order parameter in sort function
dt = np.dtype([('name', 'S10'),('age', int)])
a = np.array([("raju",21),("anil",25),("ravi", 17), ("amar",27)], dtype = dt)
print 'Our array is:'
print a
print '\n'
print 'Order by name:'
print np.sort(a, order = 'name')
它将产生以下输出 -
Our array is:
[[3 7]
[9 1]]
Applying sort() function:
[[3 7]
[1 9]]
Sort along axis 0:
[[3 1]
[9 7]]
Our array is:
[('raju', 21) ('anil', 25) ('ravi', 17) ('amar', 27)]
Order by name:
[('amar', 27) ('anil', 25) ('raju', 21) ('ravi', 17)]
numpy.argsort()
numpy.argsort()函数对输入数组执行间接排序,沿给定轴并使用指定的排序类型返回数据索引数组。 此索引数组用于构造排序数组。
例子 (Example)
import numpy as np
x = np.array([3, 1, 2])
print 'Our array is:'
print x
print '\n'
print 'Applying argsort() to x:'
y = np.argsort(x)
print y
print '\n'
print 'Reconstruct original array in sorted order:'
print x[y]
print '\n'
print 'Reconstruct the original array using loop:'
for i in y:
print x[i],
它将产生以下输出 -
Our array is:
[3 1 2]
Applying argsort() to x:
[1 2 0]
Reconstruct original array in sorted order:
[1 2 3]
Reconstruct the original array using loop:
1 2 3
numpy.lexsort()
function使用一系列键执行间接排序。 密钥可以看作电子表格中的一列。 该函数返回索引数组,使用该数组可以获取排序数据。 请注意,最后一个键恰好是排序的主键。
例子 (Example)
import numpy as np
nm = ('raju','anil','ravi','amar')
dv = ('f.y.', 's.y.', 's.y.', 'f.y.')
ind = np.lexsort((dv,nm))
print 'Applying lexsort() function:'
print ind
print '\n'
print 'Use this index to get sorted data:'
print [nm[i] + ", " + dv[i] for i in ind]
它将产生以下输出 -
Applying lexsort() function:
[3 1 0 2]
Use this index to get sorted data:
['amar, f.y.', 'anil, s.y.', 'raju, f.y.', 'ravi, s.y.']
NumPy模块具有许多用于在数组内搜索的函数。 可以使用找到最大值,最小值以及满足给定条件的元素的函数。
numpy.argmax() and numpy.argmin()
这两个函数分别沿给定轴返回最大和最小元素的索引。
例子 (Example)
import numpy as np
a = np.array([[30,40,70],[80,20,10],[50,90,60]])
print 'Our array is:'
print a
print '\n'
print 'Applying argmax() function:'
print np.argmax(a)
print '\n'
print 'Index of maximum number in flattened array'
print a.flatten()
print '\n'
print 'Array containing indices of maximum along axis 0:'
maxindex = np.argmax(a, axis = 0)
print maxindex
print '\n'
print 'Array containing indices of maximum along axis 1:'
maxindex = np.argmax(a, axis = 1)
print maxindex
print '\n'
print 'Applying argmin() function:'
minindex = np.argmin(a)
print minindex
print '\n'
print 'Flattened array:'
print a.flatten()[minindex]
print '\n'
print 'Flattened array along axis 0:'
minindex = np.argmin(a, axis = 0)
print minindex
print '\n'
print 'Flattened array along axis 1:'
minindex = np.argmin(a, axis = 1)
print minindex
它将产生以下输出 -
Our array is:
[[30 40 70]
[80 20 10]
[50 90 60]]
Applying argmax() function:
7
Index of maximum number in flattened array
[30 40 70 80 20 10 50 90 60]
Array containing indices of maximum along axis 0:
[1 2 0]
Array containing indices of maximum along axis 1:
[2 0 1]
Applying argmin() function:
5
Flattened array:
10
Flattened array along axis 0:
[0 1 1]
Flattened array along axis 1:
[0 2 0]
numpy.nonzero()
numpy.nonzero()函数返回输入数组中非零元素的索引。
例子 (Example)
import numpy as np
a = np.array([[30,40,0],[0,20,10],[50,0,60]])
print 'Our array is:'
print a
print '\n'
print 'Applying nonzero() function:'
print np.nonzero (a)
它将产生以下输出 -
Our array is:
[[30 40 0]
[ 0 20 10]
[50 0 60]]
Applying nonzero() function:
(array([0, 0, 1, 1, 2, 2]), array([0, 1, 1, 2, 0, 2]))
numpy.where()
where()函数返回满足给定条件的输入数组中元素的索引。
例子 (Example)
import numpy as np
x = np.arange(9.).reshape(3, 3)
print 'Our array is:'
print x
print 'Indices of elements > 3'
y = np.where(x > 3)
print y
print 'Use these indices to get elements satisfying the condition'
print x[y]
它将产生以下输出 -
Our array is:
[[ 0. 1. 2.]
[ 3. 4. 5.]
[ 6. 7. 8.]]
Indices of elements > 3
(array([1, 1, 2, 2, 2]), array([1, 2, 0, 1, 2]))
Use these indices to get elements satisfying the condition
[ 4. 5. 6. 7. 8.]
numpy.extract()
extract()函数返回满足任何条件的元素。
import numpy as np
x = np.arange(9.).reshape(3, 3)
print 'Our array is:'
print x
# define a condition
condition = np.mod(x,2) == 0
print 'Element-wise value of condition'
print condition
print 'Extract elements using condition'
print np.extract(condition, x)
它将产生以下输出 -
Our array is:
[[ 0. 1. 2.]
[ 3. 4. 5.]
[ 6. 7. 8.]]
Element-wise value of condition
[[ True False True]
[False True False]
[ True False True]]
Extract elements using condition
[ 0. 2. 4. 6. 8.]
NumPy - Byte Swapping
我们已经看到存储在计算机内存中的数据取决于CPU使用的体系结构。 它可能是little-endian(最低有效存储在最小地址中)或big-endian(最小地址中最高有效字节)。
numpy.ndarray.byteswap()
numpy.ndarray.byteswap()函数在两个表示之间切换:bigendian和little-endian。
import numpy as np
a = np.array([1, 256, 8755], dtype = np.int16)
print 'Our array is:'
print a
print 'Representation of data in memory in hexadecimal form:'
print map(hex,a)
# byteswap() function swaps in place by passing True parameter
print 'Applying byteswap() function:'
print a.byteswap(True)
print 'In hexadecimal form:'
print map(hex,a)
# We can see the bytes being swapped
它将产生以下输出 -
Our array is:
[1 256 8755]
Representation of data in memory in hexadecimal form:
['0x1', '0x100', '0x2233']
Applying byteswap() function:
[256 1 13090]
In hexadecimal form:
['0x100', '0x1', '0x3322']
NumPy - Copies & Views
在执行函数时,其中一些函数返回输入数组的副本,而一些函数返回视图。 当内容物理存储在另一个位置时,称为Copy 。 另一方面,如果提供相同内存内容的不同视图,我们将其称为View 。
没有副本
简单赋值不会生成数组对象的副本。 相反,它使用原始数组的相同id()来访问它。 id()返回Python对象的通用标识符,类似于C中的指针。
此外,任何一方面的任何变化都会反映在另一方面。 例如,改变一个的形状也会改变另一个的形状。
例子 (Example)
import numpy as np
a = np.arange(6)
print 'Our array is:'
print a
print 'Applying id() function:'
print id(a)
print 'a is assigned to b:'
b = a
print b
print 'b has same id():'
print id(b)
print 'Change shape of b:'
b.shape = 3,2
print b
print 'Shape of a also gets changed:'
print a
它将产生以下输出 -
Our array is:
[0 1 2 3 4 5]
Applying id() function:
139747815479536
a is assigned to b:
[0 1 2 3 4 5]
b has same id():
139747815479536
Change shape of b:
[[0 1]
[2 3]
[4 5]]
Shape of a also gets changed:
[[0 1]
[2 3]
[4 5]]
查看或浅拷贝
NumPy有ndarray.view()方法,它是一个新的数组对象,它查看原始数组的相同数据。 与前面的情况不同,新阵列的尺寸变化不会改变原始尺寸。
例子 (Example)
import numpy as np
# To begin with, a is 3X2 array
a = np.arange(6).reshape(3,2)
print 'Array a:'
print a
print 'Create view of a:'
b = a.view()
print b
print 'id() for both the arrays are different:'
print 'id() of a:'
print id(a)
print 'id() of b:'
print id(b)
# Change the shape of b. It does not change the shape of a
b.shape = 2,3
print 'Shape of b:'
print b
print 'Shape of a:'
print a
它将产生以下输出 -
Array a:
[[0 1]
[2 3]
[4 5]]
Create view of a:
[[0 1]
[2 3]
[4 5]]
id() for both the arrays are different:
id() of a:
140424307227264
id() of b:
140424151696288
Shape of b:
[[0 1 2]
[3 4 5]]
Shape of a:
[[0 1]
[2 3]
[4 5]]
切片的数组创建一个视图。
例子 (Example)
import numpy as np
a = np.array([[10,10], [2,3], [4,5]])
print 'Our array is:'
print a
print 'Create a slice:'
s = a[:, :2]
print s
它将产生以下输出 -
Our array is:
[[10 10]
[ 2 3]
[ 4 5]]
Create a slice:
[[10 10]
[ 2 3]
[ 4 5]]
深拷贝
ndarray.copy()函数创建一个深层副本。 它是数组及其数据的完整副本,不与原始数组共享。
例子 (Example)
import numpy as np
a = np.array([[10,10], [2,3], [4,5]])
print 'Array a is:'
print a
print 'Create a deep copy of a:'
b = a.copy()
print 'Array b is:'
print b
#b does not share any memory of a
print 'Can we write b is a'
print b is a
print 'Change the contents of b:'
b[0,0] = 100
print 'Modified array b:'
print b
print 'a remains unchanged:'
print a
它将产生以下输出 -
Array a is:
[[10 10]
[ 2 3]
[ 4 5]]
Create a deep copy of a:
Array b is:
[[10 10]
[ 2 3]
[ 4 5]]
Can we write b is a
False
Change the contents of b:
Modified array b:
[[100 10]
[ 2 3]
[ 4 5]]
a remains unchanged:
[[10 10]
[ 2 3]
[ 4 5]]
NumPy - Matrix Library
NumPy包中包含一个Matrix库numpy.matlib 。 该模块具有返回矩阵而不是ndarray对象的函数。
matlib.empty()
matlib.empty()函数返回一个新矩阵而不初始化条目。 该函数采用以下参数。
numpy.matlib.empty(shape, dtype, order)
Where,
Sr.No. | 参数和描述 |
---|---|
1 | shape int或tuple定义新矩阵的形状 |
2 | Dtype 可选的。 输出的数据类型 |
3 | order C或F. |
例子 (Example)
import numpy.matlib
import numpy as np
print np.matlib.empty((2,2))
# filled with random data
它将产生以下输出 -
[[ 2.12199579e-314, 4.24399158e-314]
[ 4.24399158e-314, 2.12199579e-314]]
numpy.matlib.zeros()
此函数返回填充零的矩阵。
import numpy.matlib
import numpy as np
print np.matlib.zeros((2,2))
它将产生以下输出 -
[[ 0. 0.]
[ 0. 0.]]
numpy.matlib.ones()
此函数返回填充1s的矩阵。
import numpy.matlib
import numpy as np
print np.matlib.ones((2,2))
它将产生以下输出 -
[[ 1. 1.]
[ 1. 1.]]
numpy.matlib.eye()
此函数返回一个矩阵,沿对角线元素为1,其他地方为零。 该函数采用以下参数。
numpy.matlib.eye(n, M,k, dtype)
Where,
Sr.No. | 参数和描述 |
---|---|
1 | n 结果矩阵中的行数 |
2 | M 列数,默认为n |
3 | k 对角线指数 |
4 | dtype 输出的数据类型 |
例子 (Example)
import numpy.matlib
import numpy as np
print np.matlib.eye(n = 3, M = 4, k = 0, dtype = float)
它将产生以下输出 -
[[ 1. 0. 0. 0.]
[ 0. 1. 0. 0.]
[ 0. 0. 1. 0.]]
numpy.matlib.identity()
numpy.matlib.identity()函数返回给定大小的Identity矩阵。 单位矩阵是一个方形矩阵,所有对角元素都为1。
import numpy.matlib
import numpy as np
print np.matlib.identity(5, dtype = float)
它将产生以下输出 -
[[ 1. 0. 0. 0. 0.]
[ 0. 1. 0. 0. 0.]
[ 0. 0. 1. 0. 0.]
[ 0. 0. 0. 1. 0.]
[ 0. 0. 0. 0. 1.]]
numpy.matlib.rand()
numpy.matlib.rand()函数返回给定大小的矩阵,其中填充了随机值。
例子 (Example)
import numpy.matlib
import numpy as np
print np.matlib.rand(3,3)
它将产生以下输出 -
[[ 0.82674464 0.57206837 0.15497519]
[ 0.33857374 0.35742401 0.90895076]
[ 0.03968467 0.13962089 0.39665201]]
Note ,矩阵总是二维的,而ndarray是n维数组。 两个对象都是可互换的。
例子 (Example)
import numpy.matlib
import numpy as np
i = np.matrix('1,2;3,4')
print i
它将产生以下输出 -
[[1 2]
[3 4]]
例子 (Example)
import numpy.matlib
import numpy as np
j = np.asarray(i)
print j
它将产生以下输出 -
[[1 2]
[3 4]]
例子 (Example)
import numpy.matlib
import numpy as np
k = np.asmatrix (j)
print k
它将产生以下输出 -
[[1 2]
[3 4]]
NumPy - Linear Algebra
NumPy包中包含numpy.linalg模块,该模块提供线性代数所需的所有功能。 下表描述了该模块中的一些重要功能。
Sr.No. | 功能说明 |
---|---|
1 | dot 两个数组的点积 |
2 | vdot 两个载体的点积 |
3 | inner 两个阵列的内在产品 |
4 | matmul 两个数组的矩阵乘积 |
5 | determinant 计算数组的行列式 |
6 | solve 求解线性矩阵方程 |
7 | inv 找到矩阵的乘法逆 |
NumPy - Matplotlib
Matplotlib是Python的绘图库。 它与NumPy一起使用,为MatLab提供了一个有效的开源替代环境。 它也可以用于PyQt和wxPython等图形工具包。
Matplotlib模块最初由John D. Hunter编写。 自2012年以来,Michael Droettboom是首席开发人员。 目前,Matplotlib ver。 1.5.1是可用的稳定版本。 该软件包以二进制分发形式提供,也可在www.matplotlib.org上以源代码形式提供。
通常,通过添加以下语句将包导入Python脚本 -
from matplotlib import pyplot as plt
这里pyplot()是matplotlib库中最重要的函数,用于绘制2D数据。 以下脚本绘制了等式y = 2x + 5
例子 (Example)
import numpy as np
from matplotlib import pyplot as plt
x = np.arange(1,11)
y = 2 * x + 5
plt.title("Matplotlib demo")
plt.xlabel("x axis caption")
plt.ylabel("y axis caption")
plt.plot(x,y)
plt.show()
从np.arange() function创建ndarray对象x作为x axis的值。 y axis的相应值存储在另一个ndarray object y 。 使用matplotlib包的plotlot子模块的plot()函数绘制这些值。
图形表示由show()函数show() 。
上面的代码应该产生以下输出 -
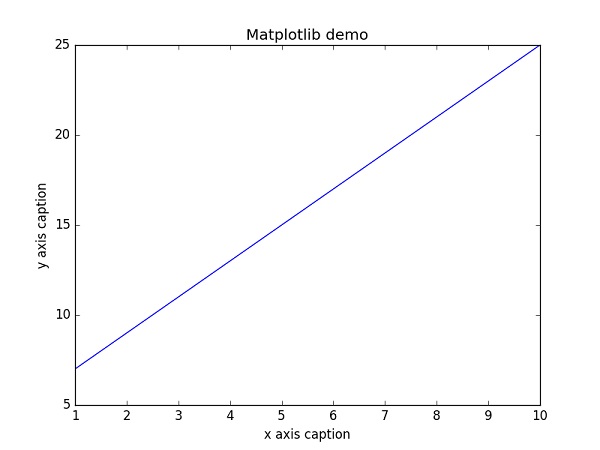
可以通过向plot()函数添加格式字符串来离散显示值,而不是线性图。 可以使用以下格式化字符。
Sr.No. | 字符和描述 |
---|---|
1 | '-' 实线风格 |
2 | '--' 虚线风格 |
3 | '-.' 短划线风格 |
4 | ':' 虚线样式 |
5 | '.' 点标记 |
6 | ',' 像素标记 |
7 | 'o' 圆形标记 |
8 | 'v' Triangle_down标记 |
9 | '^' Triangle_up标记 |
10 | '《' Triangle_left标记 |
11 | '》' Triangle_right标记 |
12 | '1' Tri_down标记 |
13 | '2' Tri_up标记 |
14 | '3' Tri_left标记 |
15 | '4' Tri_right标记 |
16 | 's' 方形标记 |
17 | 'p' 五角大楼标记 |
18 | '*' 明星标记 |
19 | 'h' Hexagon1标记 |
20 | 'H' Hexagon2标记 |
21 | '+' 再加上标记 |
22 | 'x' X标记 |
23 | 'D' 钻石标记 |
24 | 'd' Thin_diamond标记 |
25 | '|' Vline标记 |
26 | '_' Hline标记 |
还定义了以下颜色缩写。
字符 | 颜色 |
---|---|
'b' | Blue |
'g' | Green |
'r' | Red |
'c' | Cyan |
'm' | Magenta |
'y' | Yellow |
'k' | Black |
'w' | White |
要显示表示点的圆,而不是上例中的线,请使用“ob”作为plot()函数中的格式字符串。
例子 (Example)
import numpy as np
from matplotlib import pyplot as plt
x = np.arange(1,11)
y = 2 * x + 5
plt.title("Matplotlib demo")
plt.xlabel("x axis caption")
plt.ylabel("y axis caption")
plt.plot(x,y,"ob")
plt.show()
上面的代码应该产生以下输出 -
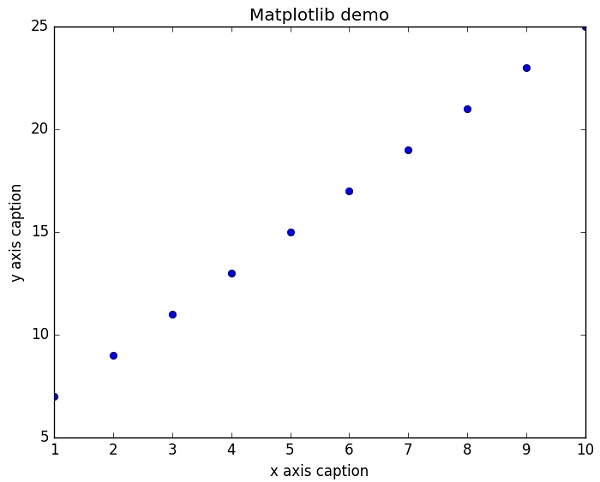
正弦波图
以下脚本使用matplotlib生成sine wave plot 。
例子 (Example)
import numpy as np
import matplotlib.pyplot as plt
# Compute the x and y coordinates for points on a sine curve
x = np.arange(0, 3 * np.pi, 0.1)
y = np.sin(x)
plt.title("sine wave form")
# Plot the points using matplotlib
plt.plot(x, y)
plt.show()
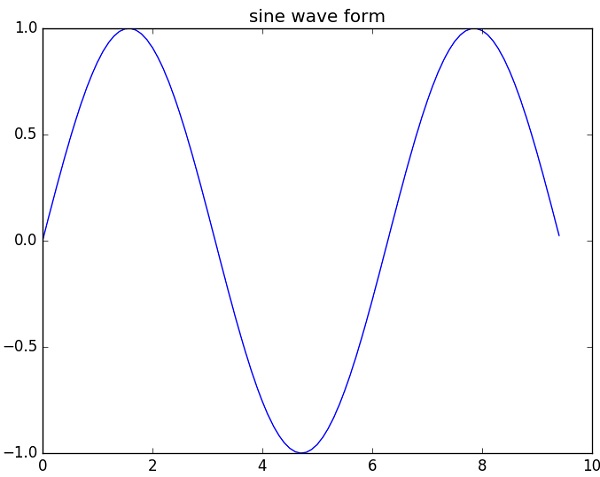
subplot()
subplot()函数允许您在同一图中绘制不同的东西。 在以下脚本中,绘制了sine和cosine values 。
例子 (Example)
import numpy as np
import matplotlib.pyplot as plt
# Compute the x and y coordinates for points on sine and cosine curves
x = np.arange(0, 3 * np.pi, 0.1)
y_sin = np.sin(x)
y_cos = np.cos(x)
# Set up a subplot grid that has height 2 and width 1,
# and set the first such subplot as active.
plt.subplot(2, 1, 1)
# Make the first plot
plt.plot(x, y_sin)
plt.title('Sine')
# Set the second subplot as active, and make the second plot.
plt.subplot(2, 1, 2)
plt.plot(x, y_cos)
plt.title('Cosine')
# Show the figure.
plt.show()
上面的代码应该产生以下输出 -
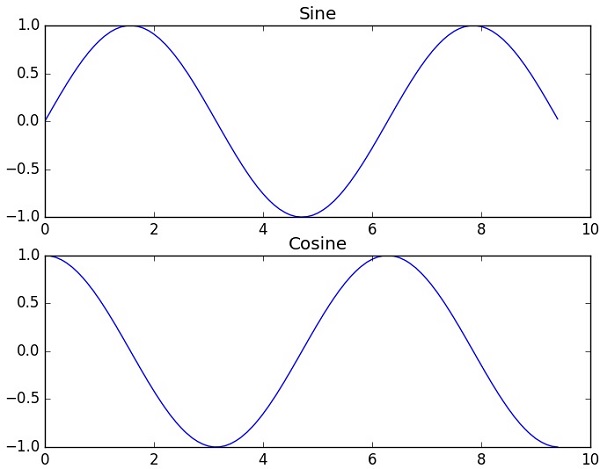
bar()
pyplot submodule提供bar()函数来生成条形图。 以下示例生成两组x和y数组的条形图。
例子 (Example)
from matplotlib import pyplot as plt
x = [5,8,10]
y = [12,16,6]
x2 = [6,9,11]
y2 = [6,15,7]
plt.bar(x, y, align = 'center')
plt.bar(x2, y2, color = 'g', align = 'center')
plt.title('Bar graph')
plt.ylabel('Y axis')
plt.xlabel('X axis')
plt.show()
此代码应产生以下输出 -
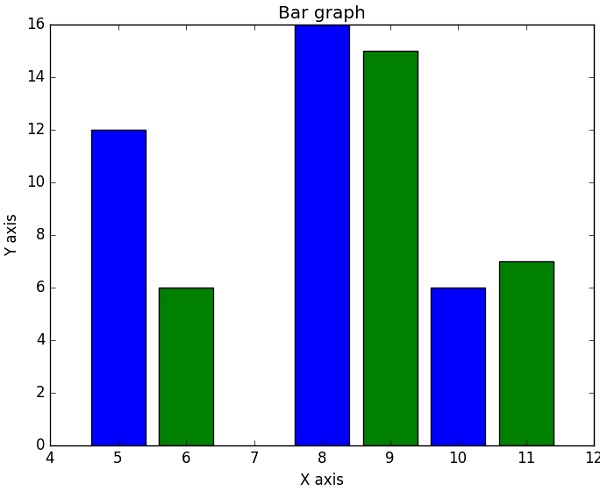
NumPy - Histogram Using Matplotlib
NumPy有一个numpy.histogram()函数,它是数据频率分布的图形表示。 相等水平尺寸的矩形对应于称为bin类间隔和对应于频率的variable height 。
numpy.histogram()
numpy.histogram()函数将输入数组和bin作为两个参数。 bin数组中的连续元素充当每个bin的边界。
import numpy as np
a = np.array([22,87,5,43,56,73,55,54,11,20,51,5,79,31,27])
np.histogram(a,bins = [0,20,40,60,80,100])
hist,bins = np.histogram(a,bins = [0,20,40,60,80,100])
print hist
print bins
它将产生以下输出 -
[3 4 5 2 1]
[0 20 40 60 80 100]
plt()
Matplotlib可以将直方图的这种数字表示转换为图形。 pyplot子模块的plt() function将包含数据和bin数组的数组作为参数并转换为直方图。
from matplotlib import pyplot as plt
import numpy as np
a = np.array([22,87,5,43,56,73,55,54,11,20,51,5,79,31,27])
plt.hist(a, bins = [0,20,40,60,80,100])
plt.title("histogram")
plt.show()
它应该产生以下输出 -
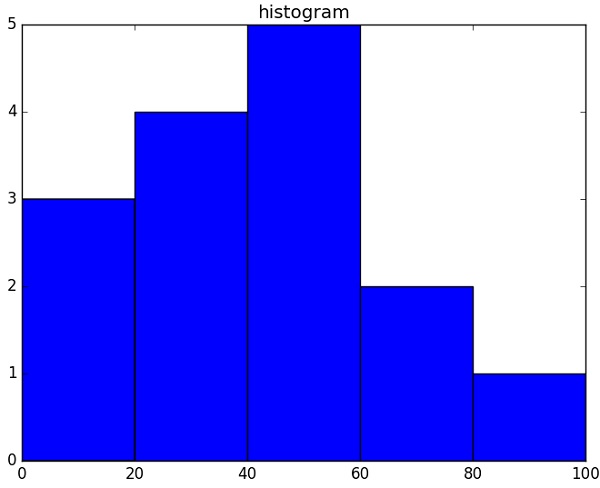
I/O with NumPy
ndarray对象可以保存到磁盘文件并从磁盘文件加载。 可用的IO功能是 -
load()和save()函数处理/ numPy二进制文件(扩展名为npy )
loadtxt()和savetxt()函数处理普通文本文件
NumPy为ndarray对象引入了一种简单的文件格式。 此.npy文件存储在磁盘文件中重建ndarray所需的数据,形状,dtype和其他信息,以便即使文件位于具有不同体系结构的另一台计算机上,也可以正确检索该数组。
numpy.save()
numpy.save()文件将输入数组存储在具有npy扩展名的磁盘文件中。
import numpy as np
a = np.array([1,2,3,4,5])
np.save('outfile',a)
要从outfile.npy重建数组,请使用load()函数。
import numpy as np
b = np.load('outfile.npy')
print b
它将产生以下输出 -
array([1, 2, 3, 4, 5])
save()和load()函数接受另一个布尔参数allow_pickles 。 Python中的pickle用于在保存到磁盘文件或从磁盘文件读取之前序列化和反序列化对象。
savetxt()
以简单文本文件格式存储和检索数组数据是使用savetxt()和loadtxt()函数完成的。
例子 (Example)
import numpy as np
a = np.array([1,2,3,4,5])
np.savetxt('out.txt',a)
b = np.loadtxt('out.txt')
print b
它将产生以下输出 -
[ 1. 2. 3. 4. 5.]
savetxt()和loadtxt()函数接受其他可选参数,如页眉,页脚和分隔符。