Cylinder
圆柱体是闭合的固体,其具有通过弯曲表面连接的两个平行(大部分为圆形)的基部。
它由两个参数描述,即 - 圆形底座的radius和圆柱体的height ,如下图所示 -
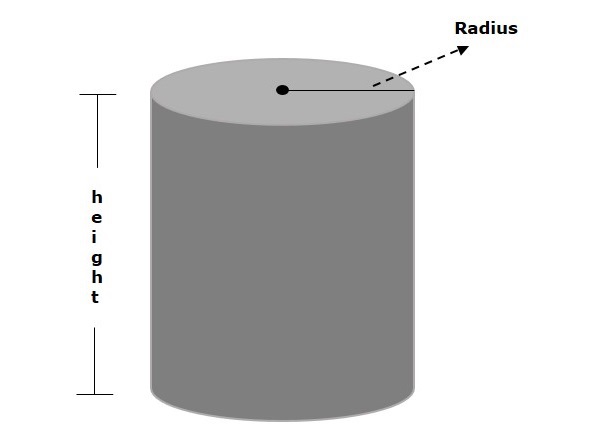
在JavaFX中,圆柱体由名为Cylinder的类表示。 该类属于包javafx.scene.shape 。 通过实例化此类,您可以在JavaFX中创建柱面节点。
该类具有double数据类型的2个属性,即 -
height - 气缸的高度。
radius - 圆柱的半径。
要绘制圆柱体,需要通过将值传递给此类的构造函数来将值传递给这些属性。 这可以在实例化时以相同的顺序完成,如以下程序所示 -
Cylinder cylinder = new Cylinder(radius, height);
或者,通过使用他们各自的setter方法如下 -
setRadius(value);
setHeight(value);
绘制3D圆柱的步骤
要在JavaFX中绘制圆柱体(3D),请按照下面给出的步骤操作。
第1步:创建一个类
创建一个Java类并继承包javafx.application的Application类,并实现javafx.application的start()方法,如下所示 -
public class ClassName extends Application {
@Override
public void start(Stage primaryStage) throws Exception {
}
}
第2步:创建一个圆柱体
您可以通过实例javafx.scene.shape为Cylinder的类来在JavaFX中创建一个Cylinder,该类属于一个包javafx.scene.shape 。 您可以按如下方式实例化此类 -
//Creating an object of the Cylinder class
Cylinder cylinder = new Cylinder();
第3步:将属性设置为圆柱体
使用各自的设定器设置气缸的height和radius ,如下所示。
//Setting the properties of the Cylinder
cylinder.setHeight(300.0f);
cylinder.setRadius(100.0f);
第4步:创建组对象
在start()方法中,通过实例javafx.scene为Group的类来创建组对象,该类属于包javafx.scene 。
将上一步中创建的Cylinder(节点)对象作为参数传递给Group类的构造函数。 这应该是为了将它添加到组中,如下所示 -
Group root = new Group(cylinder);
第5步:创建场景对象
通过实例javafx.scene为Scene的类来创建一个Scene,该类属于包javafx.scene 。 在此类中,传递上一步中创建的Group对象( root )。
除了根对象之外,您还可以传递两个表示屏幕高度和宽度的双参数以及Group类的对象,如下所示。
Scene scene = new Scene(group ,600, 300);
第6步:设置舞台的标题
您可以使用Stage类的setTitle()方法将标题设置为Stage 。 此primaryStage是Stage对象,它作为参数传递给场景类的start方法。
使用primaryStage对象,将场景标题设置为Sample Application ,如下所示。
primaryStage.setTitle("Sample Application");
第7步:将场景添加到舞台
您可以使用名为Stage的类的方法setScene()将Scene对象添加到Stage 。 使用此方法添加前面步骤中准备的Scene对象,如下所示。
primaryStage.setScene(scene);
第8步:显示舞台的内容
使用Stage类的名为show()的方法show()场景的内容,如下所示。
primaryStage.show();
第9步:启动应用程序
通过从main方法调用Application类的静态方法launch()来启动JavaFX应用程序,如下所示。
public static void main(String args[]){
launch(args);
}
例子 (Example)
以下程序显示了如何使用JavaFX生成Cylinder。 将此代码保存在名为CylinderExample.java的文件中。
import javafx.application.Application;
import javafx.scene.Group;
import javafx.scene.Scene;
import javafx.scene.shape.CullFace;
import javafx.scene.shape.Cylinder;
import javafx.stage.Stage;
public class CylinderExample extends Application {
@Override
public void start(Stage stage) {
//Drawing a Cylinder
Cylinder cylinder = new Cylinder();
//Setting the properties of the Cylinder
cylinder.setHeight(300.0f);
cylinder.setRadius(100.0f);
//Creating a Group object
Group root = new Group(cylinder);
//Creating a scene object
Scene scene = new Scene(root, 600, 300);
//Setting title to the Stage
stage.setTitle("Drawing a cylinder");
//Adding scene to the stage
stage.setScene(scene);
//Displaying the contents of the stage
stage.show();
}
public static void main(String args[]){
launch(args);
}
}
使用以下命令从命令提示符编译并执行保存的java文件。
javac CylinderExample.java
java CylinderExample
执行时,上述程序生成一个显示Cylinder的JavaFX窗口,如下所示。
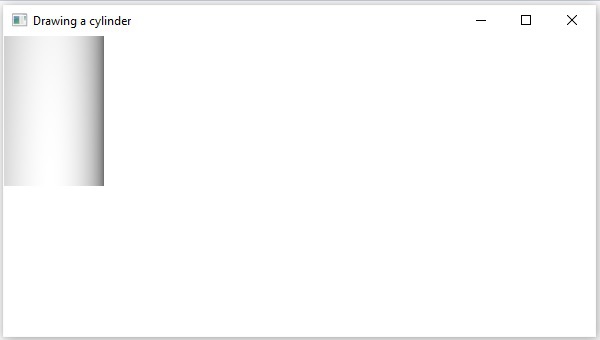