JavaFX - UI控件( UI Controls)
每个用户界面都考虑以下三个主要方面 -
UI elements - 这些是用户最终看到并与之交互的核心可视元素。 JavaFX提供了大量广泛使用的常用元素列表,从基本到复杂,我们将在本教程中介绍。
Layouts - 它们定义了如何在屏幕上组织UI元素,并为GUI(图形用户界面)提供最终外观。 这部分将在布局章节中介绍。
Behavior - 这些是用户与UI元素交互时发生的事件。 这部分将在“事件处理”一章中介绍。
JavaFX在包javafx.scene.control提供了几个类。 为了创建各种GUI组件(控件),JavaFX支持多种控件,如日期选择器,按钮文本字段等。
每个控件由一个类表示; 您可以通过实例化其各自的类来创建控件。
以下是使用JavaFX设计GUI时常用控件的列表。
S.No | 控制和描述 |
---|---|
1 | Label Label对象是用于放置文本的组件。 |
2 | Button 该类创建一个带标签的按钮。 |
3 | ColorPicker ColorPicker提供了一个控件窗格,旨在允许用户操作和选择颜色。 |
4 | CheckBox CheckBox是一个图形组件,可以处于on(true)或off(false)状态。 |
5 | RadioButton RadioButton类是一个图形组件,可以在组中处于ON(true)或OFF(false)状态。 |
6 | ListView ListView组件向用户显示文本项的滚动列表。 |
7 | TextField TextField对象是一个文本组件,允许编辑单行文本。 |
8 | PasswordField PasswordField对象是专门用于输入密码的文本组件。 |
9 | Scrollbar Scrollbar控件表示滚动条组件,以便用户可以从值范围中进行选择。 |
10 | FileChooser FileChooser控件表示用户可以从中选择文件的对话窗口。 |
11 | ProgressBar 随着任务进展完成,进度条显示任务的完成百分比。 |
12 | Slider 滑块允许用户通过在有界区间内滑动旋钮以图形方式选择值。 |
例子 (Example)
以下程序是在JavaFX中显示登录页面的示例。 在这里,我们使用控件label, text field, password field和button 。
将此代码保存在名为LoginPage.java的文件中。
import javafx.application.Application;
import static javafx.application.Application.launch;
import javafx.geometry.Insets;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.PasswordField;
import javafx.scene.layout.GridPane;
import javafx.scene.text.Text;
import javafx.scene.control.TextField;
import javafx.stage.Stage;
public class LoginPage extends Application {
@Override
public void start(Stage stage) {
//creating label email
Text text1 = new Text("Email");
//creating label password
Text text2 = new Text("Password");
//Creating Text Filed for email
TextField textField1 = new TextField();
//Creating Text Filed for password
PasswordField textField2 = new PasswordField();
//Creating Buttons
Button button1 = new Button("Submit");
Button button2 = new Button("Clear");
//Creating a Grid Pane
GridPane gridPane = new GridPane();
//Setting size for the pane
gridPane.setMinSize(400, 200);
//Setting the padding
gridPane.setPadding(new Insets(10, 10, 10, 10));
//Setting the vertical and horizontal gaps between the columns
gridPane.setVgap(5);
gridPane.setHgap(5);
//Setting the Grid alignment
gridPane.setAlignment(Pos.CENTER);
//Arranging all the nodes in the grid
gridPane.add(text1, 0, 0);
gridPane.add(textField1, 1, 0);
gridPane.add(text2, 0, 1);
gridPane.add(textField2, 1, 1);
gridPane.add(button1, 0, 2);
gridPane.add(button2, 1, 2);
//Styling nodes
button1.setStyle("-fx-background-color: darkslateblue; -fx-text-fill: white;");
button2.setStyle("-fx-background-color: darkslateblue; -fx-text-fill: white;");
text1.setStyle("-fx-font: normal bold 20px 'serif' ");
text2.setStyle("-fx-font: normal bold 20px 'serif' ");
gridPane.setStyle("-fx-background-color: BEIGE;");
//Creating a scene object
Scene scene = new Scene(gridPane);
//Setting title to the Stage
stage.setTitle("CSS Example");
//Adding scene to the stage
stage.setScene(scene);
//Displaying the contents of the stage
stage.show();
}
public static void main(String args[]){
launch(args);
}
}
使用以下命令从命令提示符编译并执行保存的java文件。
javac LoginPage.java
java LoginPage
执行时,上面的程序生成一个JavaFX窗口,如下所示。
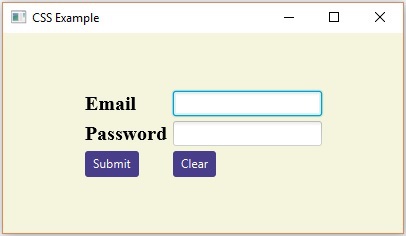
以下程序是注册表单的示例,它演示了JavaFX中的控件,如Date Picker, Radio Button, Toggle Button, Check Box, List View, Choice List,等。
将此代码保存在名为Registration.java的文件中。
import javafx.application.Application;
import javafx.collections.FXCollections;
import javafx.collections.ObservableList;
import javafx.geometry.Insets;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.CheckBox;
import javafx.scene.control.ChoiceBox;
import javafx.scene.control.DatePicker;
import javafx.scene.control.ListView;
import javafx.scene.control.RadioButton;
import javafx.scene.layout.GridPane;
import javafx.scene.text.Text;
import javafx.scene.control.TextField;
import javafx.scene.control.ToggleGroup;
import javafx.scene.control.ToggleButton;
import javafx.stage.Stage;
public class Registration extends Application {
@Override
public void start(Stage stage) {
//Label for name
Text nameLabel = new Text("Name");
//Text field for name
TextField nameText = new TextField();
//Label for date of birth
Text dobLabel = new Text("Date of birth");
//date picker to choose date
DatePicker datePicker = new DatePicker();
//Label for gender
Text genderLabel = new Text("gender");
//Toggle group of radio buttons
ToggleGroup groupGender = new ToggleGroup();
RadioButton maleRadio = new RadioButton("male");
maleRadio.setToggleGroup(groupGender);
RadioButton femaleRadio = new RadioButton("female");
femaleRadio.setToggleGroup(groupGender);
//Label for reservation
Text reservationLabel = new Text("Reservation");
//Toggle button for reservation
ToggleButton Reservation = new ToggleButton();
ToggleButton yes = new ToggleButton("Yes");
ToggleButton no = new ToggleButton("No");
ToggleGroup groupReservation = new ToggleGroup();
yes.setToggleGroup(groupReservation);
no.setToggleGroup(groupReservation);
//Label for technologies known
Text technologiesLabel = new Text("Technologies Known");
//check box for education
CheckBox javaCheckBox = new CheckBox("Java");
javaCheckBox.setIndeterminate(false);
//check box for education
CheckBox dotnetCheckBox = new CheckBox("DotNet");
javaCheckBox.setIndeterminate(false);
//Label for education
Text educationLabel = new Text("Educational qualification");
//list View for educational qualification
ObservableList<String> names = FXCollections.observableArrayList(
"Engineering", "MCA", "MBA", "Graduation", "MTECH", "Mphil", "Phd");
ListView<String> educationListView = new ListView<String>(names);
//Label for location
Text locationLabel = new Text("location");
//Choice box for location
ChoiceBox locationchoiceBox = new ChoiceBox();
locationchoiceBox.getItems().addAll
("Hyderabad", "Chennai", "Delhi", "Mumbai", "Vishakhapatnam");
//Label for register
Button buttonRegister = new Button("Register");
//Creating a Grid Pane
GridPane gridPane = new GridPane();
//Setting size for the pane
gridPane.setMinSize(500, 500);
//Setting the padding
gridPane.setPadding(new Insets(10, 10, 10, 10));
//Setting the vertical and horizontal gaps between the columns
gridPane.setVgap(5);
gridPane.setHgap(5);
//Setting the Grid alignment
gridPane.setAlignment(Pos.CENTER);
//Arranging all the nodes in the grid
gridPane.add(nameLabel, 0, 0);
gridPane.add(nameText, 1, 0);
gridPane.add(dobLabel, 0, 1);
gridPane.add(datePicker, 1, 1);
gridPane.add(genderLabel, 0, 2);
gridPane.add(maleRadio, 1, 2);
gridPane.add(femaleRadio, 2, 2);
gridPane.add(reservationLabel, 0, 3);
gridPane.add(yes, 1, 3);
gridPane.add(no, 2, 3);
gridPane.add(technologiesLabel, 0, 4);
gridPane.add(javaCheckBox, 1, 4);
gridPane.add(dotnetCheckBox, 2, 4);
gridPane.add(educationLabel, 0, 5);
gridPane.add(educationListView, 1, 5);
gridPane.add(locationLabel, 0, 6);
gridPane.add(locationchoiceBox, 1, 6);
gridPane.add(buttonRegister, 2, 8);
//Styling nodes
buttonRegister.setStyle(
"-fx-background-color: darkslateblue; -fx-textfill: white;");
nameLabel.setStyle("-fx-font: normal bold 15px 'serif' ");
dobLabel.setStyle("-fx-font: normal bold 15px 'serif' ");
genderLabel.setStyle("-fx-font: normal bold 15px 'serif' ");
reservationLabel.setStyle("-fx-font: normal bold 15px 'serif' ");
technologiesLabel.setStyle("-fx-font: normal bold 15px 'serif' ");
educationLabel.setStyle("-fx-font: normal bold 15px 'serif' ");
locationLabel.setStyle("-fx-font: normal bold 15px 'serif' ");
//Setting the back ground color
gridPane.setStyle("-fx-background-color: BEIGE;");
//Creating a scene object
Scene scene = new Scene(gridPane);
//Setting title to the Stage
stage.setTitle("Registration Form");
//Adding scene to the stage
stage.setScene(scene);
//Displaying the contents of the stage
stage.show();
}
public static void main(String args[]){
launch(args);
}
}
使用以下命令从命令提示符编译并执行保存的java文件。
javac Registration.java
java Registration
执行时,上面的程序生成一个JavaFX窗口,如下所示。
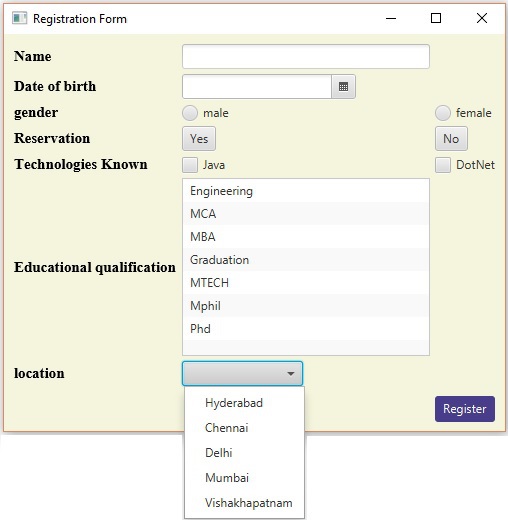