switch statement
switch语句允许测试变量与值列表的相等性。 每个值称为一个case ,并检查每个开关案例接通的变量。 以下是JavaScript中switch的语法。
switch (expression){
case condition 1: statement(s)
break;
case condition 2: statement(s)
break;
case condition n: statement(s)
break;
default: statement(s)
}
在JavaScript中,在每个switch case之后,我们必须使用break语句。 如果我们不小心忘记了break语句,那么有可能从一个switch case掉到另一个。
在CoffeeScript中切换语句
CoffeeScript通过使用switch-when-else子句的组合解决了这个问题。 这里我们有一个可选的switch表达式,后跟case语句。
每个case语句有时有两个子句。 when后跟条件, then是满足特定条件时要执行的语句集。 最后,我们有可选的else子句来保存默认条件的操作。
语法 (Syntax)
下面给出了CoffeeScript中switch语句的语法。 我们指定没有括号的表达式,我们通过维护适当的缩进来分隔case语句。
switch expression
when condition1 then statements
when condition2 then statements
when condition3 then statements
else statements
流程图 (Flow Diagram)
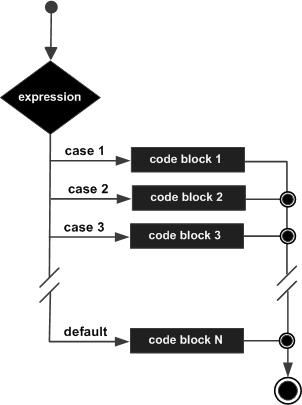
例子 (Example)
以下示例演示了CoffeeScript中switch语句的用法。 将此代码保存在名为switch_example.coffee的文件中
name="Ramu"
score=75
message = switch
when score>=75 then "Congrats your grade is A"
when score>=60 then "Your grade is B"
when score>=50 then "Your grade is C"
when score>=35 then "Your grade is D"
else "Your grade is F and you are failed in the exam"
console.log message
打开command prompt并编译.coffee文件,如下所示。
c:\> coffee -c switch_exmple.coffee
在编译时,它为您提供以下JavaScript。
// Generated by CoffeeScript 1.10.0
(function() {
var message, name, score;
name = "Ramu";
score = 75;
message = (function() {
switch (false) {
case !(score >= 75):
return "Congrats your grade is A";
case !(score >= 60):
return "Your grade is B";
case !(score >= 50):
return "Your grade is C";
case !(score >= 35):
return "Your grade is D";
default:
return "Your grade is F and you are failed in the exam";
}
})();
console.log(message);
}).call(this);
现在,再次打开command prompt并运行CoffeeScript文件 -
c:\> coffee switch_exmple.coffee
执行时,CoffeeScript文件生成以下输出。
Congrats your grade is A
when子句的多个值
我们还可以通过在switch case中使用逗号( , )分隔单个when子句来指定多个值。
例子 (Example)
以下示例显示如何通过为when子句指定多个值来编写CoffeeScript开关语句。 将此代码保存在名为switch_multiple_example.coffee的文件中
name="Ramu"
score=75
message = switch name
when "Ramu","Mohammed" then "You have passed the examination with grade A"
when "John","Julia" then "You have passed the examination with grade is B"
when "Rajan" then "Sorry you failed in the examination"
else "No result"
console.log message
打开command prompt并编译.coffee文件,如下所示。
c:\> coffee -c switch_multiple_example.coffee
在编译时,它为您提供以下JavaScript。
// Generated by CoffeeScript 1.10.0
(function() {
var message, name, score;
name = "Ramu";
score = 75;
message = (function() {
switch (name) {
case "Ramu":
case "Mohammed":
return "You have passed the examination with grade A";
case "John":
case "Julia":
return "You have passed the examination with grade is B";
case "Rajan":
return "Sorry you failed in the examination";
default:
return "No result";
}
})();
console.log(message);
}).call(this);
现在,再次打开command prompt并运行CoffeeScript文件,如下所示。
c:\> coffee switch_multiple_example.coffee
执行时,CoffeeScript文件生成以下输出。
You have passed the examination with grade A