Struts2 - 动作( Actions)
Actions是Struts2框架的核心,因为它们适用于任何MVC(模型视图控制器)框架。 每个URL都映射到一个特定的操作,该操作提供了处理来自用户的请求所必需的处理逻辑。
但该行动还有两个重要的能力。 首先,无论是JSP还是其他类型的结果,该操作在从请求到视图的数据传输中起着重要作用。 其次,该操作必须帮助框架确定哪个结果应该呈现将在请求的响应中返回的视图。
Create Action
Struts2操作的唯一要求是必须有一个noargument方法返回String或Result对象,并且必须是POJO。 如果未指定无参数方法,则默认行为是使用execute()方法。
(可选)您可以扩展ActionSupport类,该类实现六个接口,包括Action接口。 Action界面如下 -
public interface Action {
public static final String SUCCESS = "success";
public static final String NONE = "none";
public static final String ERROR = "error";
public static final String INPUT = "input";
public static final String LOGIN = "login";
public String execute() throws Exception;
}
让我们看一下Hello World示例中的action方法 -
package com.iowiki.struts2;
public class HelloWorldAction {
private String name;
public String execute() throws Exception {
return "success";
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
为了说明action方法控制视图的点,让我们对execute方法进行以下更改,并按如下方式扩展ActionSupport类 -
package com.iowiki.struts2;
import com.opensymphony.xwork2.ActionSupport;
public class HelloWorldAction extends ActionSupport {
private String name;
public String execute() throws Exception {
if ("SECRET".equals(name)) {
return SUCCESS;
} else {
return ERROR;
}
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
在这个例子中,我们在execute方法中有一些逻辑来查看name属性。 如果属性等于字符串"SECRET" ,我们返回SUCCESS作为结果,否则我们返回ERROR作为结果。 因为我们已经扩展了ActionSupport,所以我们可以使用String常量SUCCESS和ERROR。 现在,让我们修改我们的struts.xml文件,如下所示 -
<?xml version = "1.0" Encoding = "UTF-8"?>
<!DOCTYPE struts PUBLIC
"-//Apache Software Foundation//DTD Struts Configuration 2.0//EN"
"http://struts.apache.org/dtds/struts-2.0.dtd">
<struts>
<constant name = "struts.devMode" value = "true" />
<package name = "helloworld" extends = "struts-default">
<action name = "hello"
class = "com.iowiki.struts2.HelloWorldAction"
method = "execute">
<result name = "success">/HelloWorld.jsp</result>
<result name = "error">/AccessDenied.jsp</result>
</action>
</package>
</struts>
创建一个视图 (Create a View)
让我们在你的eclipse项目的WebContent文件夹中创建下面的jsp文件HelloWorld.jsp 。 为此,右键单击项目资源管理器中的WebContent文件夹,然后选择“ New 》JSP File 。 如果返回结果为SUCCESS,则会调用此文件,这是Action接口中定义的String常量“success” -
<%@ page contentType = "text/html; charset = UTF-8" %>
<%@ taglib prefix = "s" uri = "/struts-tags" %>
<html>
<head>
<title>Hello World</title>
</head>
<body>
Hello World, <s:property value = "name"/>
</body>
</html>
以下是框架将调用的文件,如果操作结果为ERROR,则等于String常量“error”。 以下是AccessDenied.jsp的内容
<%@ page contentType = "text/html; charset = UTF-8" %>
<%@ taglib prefix = "s" uri = "/struts-tags" %>
<html>
<head>
<title>Access Denied</title>
</head>
<body>
You are not authorized to view this page.
</body>
</html>
我们还需要在WebContent文件夹中创建index.jsp 。 该文件将作为初始操作URL,用户可以单击该URL以告诉Struts 2框架调用HelloWorldAction类的execute方法并呈现HelloWorld.jsp视图。
<%@ page language = "java" contentType = "text/html; charset = ISO-8859-1"
pageEncoding = "ISO-8859-1"%>
<%@ taglib prefix = "s" uri = "/struts-tags"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN"
"http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<title>Hello World</title>
</head>
<body>
<h1>Hello World From Struts2</h1>
<form action = "hello">
<label for = "name">Please enter your name</label><br/>
<input type = "text" name = "name"/>
<input type = "submit" value = "Say Hello"/>
</form>
</body>
</html>
就是这样,web.xml文件不需要更改,所以让我们使用我们在Examples章节中创建的web.xml。 现在,我们已准备好使用Struts 2框架运行Hello World应用程序。
执行应用程序
右键单击项目名称,然后单击“ Export 》 WAR文件以创建War文件。 然后在Tomcat的webapps目录中部署此WAR。 最后,启动Tomcat服务器并尝试访问URL http://localhost:8080/HelloWorldStruts2/index.jsp 。 这将为您提供以下屏幕 -
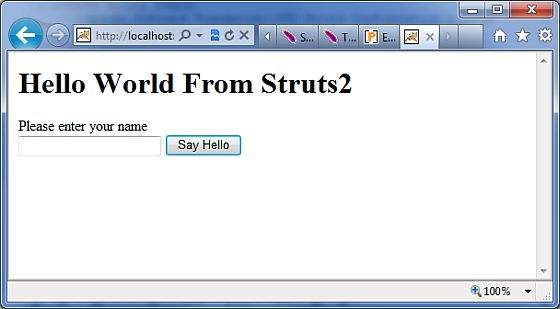
让我们输入一个单词“SECRET”,您应该看到以下页面 -
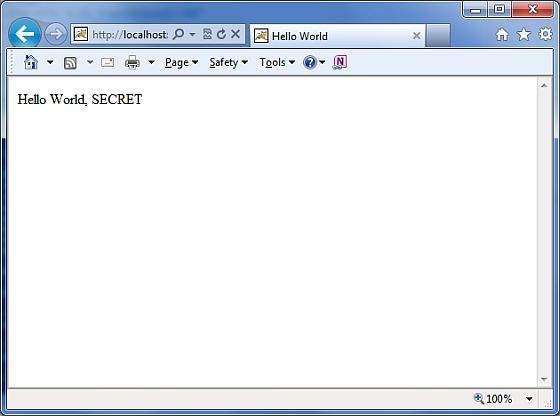
现在输入“SECRET”以外的任何单词,您应该看到以下页面 -

创建多个操作
您将经常定义多个操作来处理不同的请求并向用户提供不同的URL,因此您将定义下面定义的不同类 -
package com.iowiki.struts2;
import com.opensymphony.xwork2.ActionSupport;
class MyAction extends ActionSupport {
public static String GOOD = SUCCESS;
public static String BAD = ERROR;
}
public class HelloWorld extends ActionSupport {
...
public String execute() {
if ("SECRET".equals(name)) return MyAction.GOOD;
return MyAction.BAD;
}
...
}
public class SomeOtherClass extends ActionSupport {
...
public String execute() {
return MyAction.GOOD;
}
...
}
您将在struts.xml文件中配置以下操作,如下所示 -
<?xml version = "1.0" Encoding = "UTF-8"?>
<!DOCTYPE struts PUBLIC
"-//Apache Software Foundation//DTD Struts Configuration 2.0//EN"
"http://struts.apache.org/dtds/struts-2.0.dtd">
<struts>
<constant name = "struts.devMode" value = "true" />
<package name = "helloworld" extends = "struts-default">
<action name = "hello"
class = "com.iowiki.struts2.HelloWorld"
method = "execute">
<result name = "success">/HelloWorld.jsp</result>
<result name = "error">/AccessDenied.jsp</result>
</action>
<action name = "something"
class = "com.iowiki.struts2.SomeOtherClass"
method = "execute">
<result name = "success">/Something.jsp</result>
<result name = "error">/AccessDenied.jsp</result>
</action>
</package>
</struts>
正如您在上面的假设示例中所看到的,操作结果SUCCESS和ERROR’s是重复的。
要解决此问题,建议您创建一个包含结果结果的类。