while循环
只要给定条件为真,Python编程语言中的while循环语句就会重复执行目标语句。
语法 (Syntax)
Python编程语言中while循环的语法是 -
while expression:
statement(s)
这里, statement(s)可以是单个语句或语句块。 condition可以是任何表达式,true是任何非零值。 当条件为真时,循环迭代。
当条件变为假时,程序控制将立即传递到循环之后的行。
在Python中,在编程构造之后由相同数量的字符空格缩进的所有语句被认为是单个代码块的一部分。 Python使用缩进作为分组语句的方法。
流程图 (Flow Diagram)
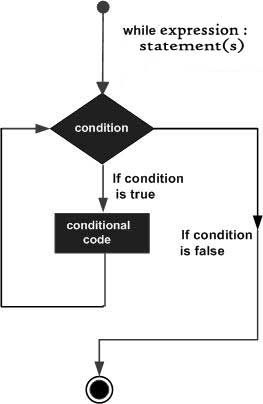
这里,while循环的关键点是循环可能永远不会运行。 当测试条件并且结果为假时,将跳过循环体并且将执行while循环之后的第一个语句。
例子 (Example)
#!/usr/bin/python
count = 0
while (count < 9):
print 'The count is:', count
count = count + 1
print "Good bye!"
执行上述代码时,会产生以下结果 -
The count is: 0
The count is: 1
The count is: 2
The count is: 3
The count is: 4
The count is: 5
The count is: 6
The count is: 7
The count is: 8
Good bye!
此处的块(由print和increment语句组成)将重复执行,直到count不再小于9.每次迭代时,将显示索引计数的当前值,然后再增加1。
无限循环 (The Infinite Loop)
如果条件永远不会变为FALSE,则循环变为无限循环。 使用while循环时必须小心,因为此条件可能永远不会解析为FALSE值。 这导致循环永远不会结束。 这种循环称为无限循环。
无限循环在客户端/服务器编程中可能很有用,其中服务器需要连续运行,以便客户端程序可以在需要时与其通信。
#!/usr/bin/python
var = 1
while var == 1 : # This constructs an infinite loop
num = raw_input("Enter a number :")
print "You entered: ", num
print "Good bye!"
执行上述代码时,会产生以下结果 -
Enter a number :20
You entered: 20
Enter a number :29
You entered: 29
Enter a number :3
You entered: 3
Enter a number between :Traceback (most recent call last):
File "test.py", line 5, in <module>
num = raw_input("Enter a number :")
KeyboardInterrupt
以上示例进入无限循环,您需要使用CTRL + C退出程序。
使用带语句的else语句
Python支持将一个else语句与循环语句相关联。
如果else语句与for循环一起使用,则在循环耗尽迭代列表时执行else语句。
如果else语句与while循环一起使用,则在条件变为false时执行else语句。
以下示例说明了else语句与while语句的组合,只要它小于5就会打印一个数字,否则会执行语句。
#!/usr/bin/python
count = 0
while count < 5:
print count, " is less than 5"
count = count + 1
else:
print count, " is not less than 5"
执行上述代码时,会产生以下结果 -
0 is less than 5
1 is less than 5
2 is less than 5
3 is less than 5
4 is less than 5
5 is not less than 5
单一套房
与if语句语法类似,如果while子句仅包含单个语句,则它可以与while标头放在同一行上。
以下是one-line while子句的语法和示例 -
#!/usr/bin/python
flag = 1
while (flag): print 'Given flag is really true!'
print "Good bye!"
最好不要尝试上面的例子,因为它进入无限循环,你需要按CTRL + C键退出。