比较两个整数(Compare two integers)
比较三个整数变量是您可以轻松编写的最简单的程序之一。 在此程序中,您可以使用scanf()函数从用户获取输入,也可以在程序本身中静态定义。
我们希望它也是一个简单的程序。 我们将一个值与其余的两个值进行比较并检查结果,并对所有变量应用相同的过程。 对于此程序,所有值都应该是不同的(唯一的)。
算法 (Algorithm)
让我们首先看看比较三个整数的分步过程应该是什么 -
START
Step 1 → Take two integer variables, say A, B& C
Step 2 → Assign values to variables
Step 3 → If A is greater than B & C, Display A is largest value
Step 4 → If B is greater than A & C, Display B is largest value
Step 5 → If C is greater than A & B, Display A is largest value
Step 6 → Otherwise, Display A, B & C are not unique values
STOP
流程图 (Flow Diagram)
我们可以绘制这个程序的流程图,如下所示 -
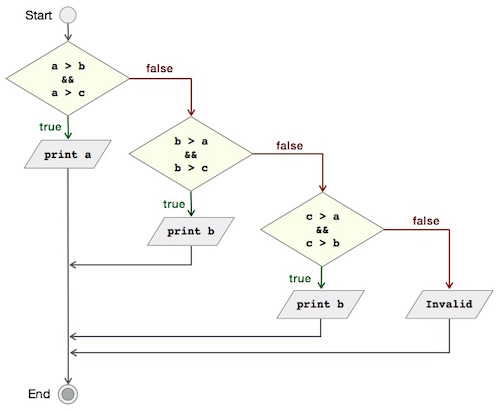
此图显示了三个if-else-if和一个else比较语句。
伪代码 (Pseudocode)
现在让我们看看这个算法的伪代码 -
procedure compare(A, B, C)
IF A is greater than B AND A is greater than C
DISPLAY "A is the largest."
ELSE IF B is greater than A AND A is greater than C
DISPLAY "B is the largest."
ELSE IF C is greater than A AND A is greater than B
DISPLAY "C is the largest."
ELSE
DISPLAY "Values not unique."
END IF
end procedure
实现 (Implementation)
现在,我们将看到该计划的实际执行情况 -
#include <stdio.h>
int main() {
int a, b, c;
a = 11;
b = 22;
c = 33;
if ( a > b && a > c )
printf("%d is the largest.", a);
else if ( b > a && b > c )
printf("%d is the largest.", b);
else if ( c > a && c > b )
printf("%d is the largest.", c);
else
printf("Values are not unique");
return 0;
}
输出 (Output)
该计划的输出应为 -
33 is the largest.