应用事件(Application Events)
JSF提供系统事件侦听器,以在JSF应用程序生命周期中执行特定于应用程序的任务。
S.No | 系统事件和描述 |
---|---|
1 | PostConstructApplicationEvent 应用程序启动时触发。 应用程序启动后可用于执行初始化任务。 |
2 | PreDestroyApplicationEvent 应用程序即将关闭时触发。 可用于在应用程序即将关闭之前执行清理任务。 |
3 | PreRenderViewEvent 在显示JSF页面之前触发。 可用于验证用户身份并提供对JSF View的受限访问。 |
可以按以下方式处理系统事件。
S.No | 技术与描述 |
---|---|
1 | SystemEventListener 实现SystemEventListener接口并在faces-config.xml中注册system-event-listener类 |
2 | Method Binding 在f:event的listener属性中传递托管bean方法的名称。 |
SystemEventListener
实现SystemEventListener接口。
public class CustomSystemEventListener implements SystemEventListener {
@Override
public void processEvent(SystemEvent event) throws
AbortProcessingException {
if(event instanceof PostConstructApplicationEvent) {
System.out.println("Application Started.
PostConstructApplicationEvent occurred!");
}
}
}
在faces-config.xml中为系统事件注册自定义系统事件侦听器。
<system-event-listener>
<system-event-listener-class>
com.iowiki.test.CustomSystemEventListener
</system-event-listener-class>
<system-event-class>
javax.faces.event.PostConstructApplicationEvent
</system-event-class>
</system-event-listener>
方法绑定
定义方法
public void handleEvent(ComponentSystemEvent event) {
data = "Hello World";
}
使用上述方法。
<f:event listener = "#{user.handleEvent}" type = "preRenderView" />
例子 Example Application
让我们创建一个测试JSF应用程序来测试JSF中的系统事件。
步 | 描述 |
---|---|
1 | 在com.iowiki.test包下创建一个名为helloworld的项目,如JSF - First Application一章中所述。 |
2 | 修改UserData.java文件,如下所述。 |
3 | 在包com.iowiki.test下创建CustomSystemEventListener.java文件。 按照以下说明修改它 |
4 | 修改home.xhtml ,如下所述。 |
5 | 在WEB-INF夹中创建faces-config.xml 。按照下面的说明进行修改。 保持其余文件不变。 |
6 | 编译并运行应用程序以确保业务逻辑按照要求运行。 |
7 | 最后,以war文件的形式构建应用程序并将其部署在Apache Tomcat Webserver中。 |
8 | 使用适当的URL启动Web应用程序,如下面的最后一步所述。 |
UserData.java
package com.iowiki.test;
import java.io.Serializable;
import javax.faces.bean.ManagedBean;
import javax.faces.bean.SessionScoped;
import javax.faces.event.ComponentSystemEvent;
@ManagedBean(name = "userData", eager = true)
@SessionScoped
public class UserData implements Serializable {
private static final long serialVersionUID = 1L;
private String data = "sample data";
public void handleEvent(ComponentSystemEvent event) {
data = "Hello World";
}
public String getData() {
return data;
}
public void setData(String data) {
this.data = data;
}
}
CustomSystemEventListener.java
package com.iowiki.test;
import javax.faces.application.Application;
import javax.faces.event.AbortProcessingException;
import javax.faces.event.PostConstructApplicationEvent;
import javax.faces.event.PreDestroyApplicationEvent;
import javax.faces.event.SystemEvent;
import javax.faces.event.SystemEventListener;
public class CustomSystemEventListener implements SystemEventListener {
@Override
public boolean isListenerForSource(Object value) {
//only for Application
return (value instanceof Application);
}
@Override
public void processEvent(SystemEvent event)
throws AbortProcessingException {
if(event instanceof PostConstructApplicationEvent) {
System.out.println("Application Started.
PostConstructApplicationEvent occurred!");
}
if(event instanceof PreDestroyApplicationEvent) {
System.out.println("PreDestroyApplicationEvent occurred.
Application is stopping.");
}
}
}
home.xhtml
<?xml version = "1.0" encoding = "UTF-8"?>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN"
"http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns = "http://www.w3.org/1999/xhtml"
xmlns:h = "http://java.sun.com/jsf/html"
xmlns:f = "http://java.sun.com/jsf/core">
<h:head>
<title>JSF tutorial</title>
</h:head>
<h:body>
<h2>Application Events Examples</h2>
<f:event listener = "#{userData.handleEvent}" type = "preRenderView" />
#{userData.data}
</h:body>
</html>
faces-config.xhtml
<?xml version = "1.0" encoding = "UTF-8"?>
<faces-config
xmlns = "http://java.sun.com/xml/ns/javaee"
xmlns:xsi = "http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation = "http://java.sun.com/xml/ns/javaee
http://java.sun.com/xml/ns/javaee/web-facesconfig_2_0.xsd"
version = "2.0">
<application>
<!-- Application Startup -->
<system-event-listener>
<system-event-listener-class>
com.iowiki.test.CustomSystemEventListener
</system-event-listener-class>
<system-event-class>
javax.faces.event.PostConstructApplicationEvent
</system-event-class>
</system-event-listener>
<!-- Before Application is to shut down -->
<system-event-listener>
<system-event-listener-class>
com.iowiki.test.CustomSystemEventListener
</system-event-listener-class>
<system-event-class>
javax.faces.event.PreDestroyApplicationEvent
</system-event-class>
</system-event-listener>
</application>
</faces-config>
一旦准备好完成所有更改,让我们像在JSF - First Application章节中那样编译和运行应用程序。 如果您的应用程序一切正常,这将产生以下结果。
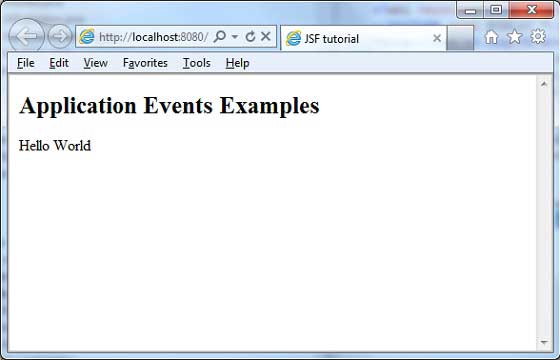
查看您的Web服务器控制台输出。 您将看到以下结果。
INFO: Deploying web application archive helloworld.war
Dec 6, 2012 8:21:44 AM com.sun.faces.config.ConfigureListener contextInitialized
INFO: Initializing Mojarra 2.1.7 (SNAPSHOT 20120206) for context '/helloworld'
Application Started. PostConstructApplicationEvent occurred!
Dec 6, 2012 8:21:46 AM com.sun.faces.config.ConfigureListener
$WebConfigResourceMonitor$Monitor <init>
INFO: Monitoring jndi:/localhost/helloworld/WEB-INF/faces-config.xml
for modifications
Dec 6, 2012 8:21:46 AM org.apache.coyote.http11.Http11Protocol start
INFO: Starting Coyote HTTP/1.1 on http-8080
Dec 6, 2012 8:21:46 AM org.apache.jk.common.ChannelSocket init
INFO: JK: ajp13 listening on /0.0.0.0:8009
Dec 6, 2012 8:21:46 AM org.apache.jk.server.JkMain start
INFO: Jk running ID = 0 time = 0/24 config = null
Dec 6, 2012 8:21:46 AM org.apache.catalina.startup.Catalina start
INFO: Server startup in 44272 ms